java发送http请求头multipart/form-data
时间: 2024-10-23 08:14:15 浏览: 34
在Java中,如果你需要发送包含文件的HTTP POST请求,并且数据类型是`multipart/form-data`,通常你会使用`java.net.HttpURLConnection`、`Apache HttpClient`或是第三方库如`Spring RestTemplate`。这里以`HttpURLConnection`为例,展示如何设置Multipart HTTP请求:
```java
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.InputStream;
import java.io.OutputStream;
import javax.servlet.http.Part;
import java.net.HttpURLConnection;
import java.net.URL;
public class MultipartRequestExample {
public static void main(String[] args) throws Exception {
URL url = new URL("http://example.com/upload");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// 设置POST请求
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW");
// 添加文件部分
Part filePart = createFilePart(new File("/path/to/your/file.jpg"), "file.jpg");
connection.setRequestProperty("Content-Length", String.valueOf(filePart.getSize()));
OutputStream os = connection.getOutputStream();
writeMultipart(os, filePart);
os.flush();
os.close();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("Upload successful");
} else {
System.out.println("Error uploading: " + responseCode);
}
connection.disconnect();
}
private static Part createFilePart(File file, String filename) throws Exception {
ByteArrayOutputStream baos = new ByteArrayOutputStream();
InputStream fis = new FileInputStream(file);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
baos.write(buffer, 0, length);
}
fis.close();
return new Part() {
@Override
public String getName() {
return filename;
}
@Override
public String getSubmittedFileName() {
return filename;
}
@Override
public InputStream getInputStream() throws IOException {
return new ByteArrayInputStream(baos.toByteArray());
}
@Override
public long getSize() {
return baos.size();
}
@Override
public String getContentType() {
return "application/octet-stream";
}
@Override
public boolean isRepeatable() {
return false;
}
@Override
public boolean isMimeMultipart() {
return false;
}
};
}
private static void writeMultipart(OutputStream os, Part part) throws Exception {
String boundary = "--" + part.getContentType().substring(part.getContentType().indexOf(';', 1) + 1);
os.write("--".getBytes());
os.write(boundary.getBytes());
os.write("\r\n".getBytes());
os.write("Content-Disposition: form-data; name=\"" + part.getName() + "\"; filename=\"" + part.getSubmittedFileName() + "\"\r\n".getBytes());
os.write("Content-Type: " + part.getContentType() + "\r\n\r\n".getBytes());
os.write(part.getInputStream().readAllBytes());
os.write("\r\n--".getBytes());
os.write(boundary.substring(2).getBytes()); // remove leading double hyphen
os.write("--\r\n".getBytes());
}
}
```
在这个示例中,我们创建了一个自定义的`Part`对象,它模拟了标准的`Part`接口,然后将这个`Part`加入到HTTP请求体中。
阅读全文
相关推荐
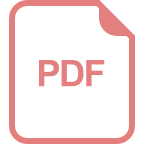
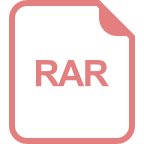
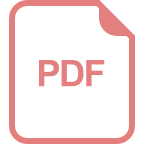
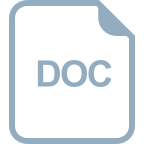
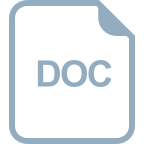













