决策树python代码实现
时间: 2023-08-04 21:09:51 浏览: 161
决策树在Python中可以使用scikit-learn库来实现。以下是一个简单的决策树的Python代码示例:
```
# 导入所需的库
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier
from sklearn import metrics
# 加载数据集
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 将数据集拆分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=1)
# 创建决策树分类器
clf = DecisionTreeClassifier()
# 在训练集上训练模型
clf.fit(X_train, y_train)
# 在测试集上进行预测
y_pred = clf.predict(X_test)
# 计算模型的准确率
print("准确率:", metrics.accuracy_score(y_test, y_pred))
```
这段代码首先导入了所需的库,包括`datasets`用于加载数据集,`train_test_split`用于将数据集拆分为训练集和测试集,`DecisionTreeClassifier`用于创建决策树分类器,以及`metrics`用于计算模型的准确率。
然后,代码加载了一个经典的鸢尾花数据集作为示例数据。接下来,将数据集拆分为训练集和测试集,其中测试集占总数据集的30%。
然后,创建了一个决策树分类器对象`clf`,并使用训练集对模型进行训练。最后,使用测试集进行预测,并计算模型的准确率。
这只是一个简单的决策树的示例,你可以根据自己的需求对代码进行修改和扩展。
阅读全文
相关推荐
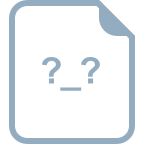
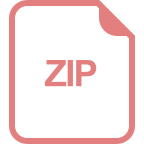
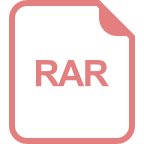
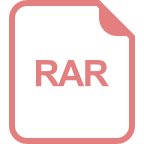
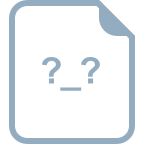
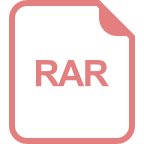
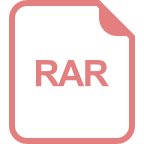
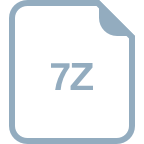
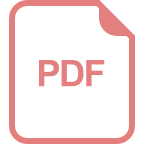
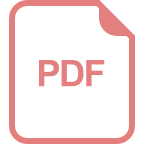
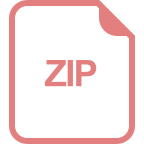
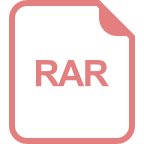
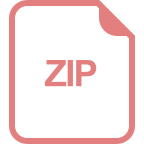
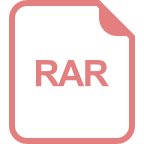