摩尔斯电码转换程序c++
时间: 2024-10-27 21:07:27 浏览: 29
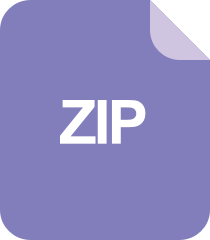
morse:将摩尔斯电码转换为文本,并将文本转换为摩尔斯电码
摩尔斯电码是一种早期的通信编码方式,通过长短音符组合代表字母、数字和其他字符。在C++中编写一个摩尔斯电码转换程序,你需要创建两个数据结构,一个用于存储字母和其对应的摩尔斯电码,另一个用于处理用户输入并显示结果。
以下是一个简单的摩尔斯电码转换程序的基本步骤:
1. 定义两个数组或字符串,一个存放英文字母及其对应电码,另一个存放电码的符号(如短划线“-”和长划线“_”)。
2. 编写函数,比如`encodeMorseCode`,接收字母作为输入,从字典中查找对应的电码,并返回它。
3. 创建另一个函数,比如`decodeMorseCode`,接收摩尔斯电码作为输入,逐个解析每个字符,并根据规则还原成字母。
4. 主程序部分接受用户输入,可能是字母或已编码的摩尔斯电码,然后调用相应的函数进行转换和显示。
```cpp
#include <iostream>
#include <string>
std::string morseAlphabet = ".-","-...","-.-.","-..",".","..-.","--.","....","..",".---","-.-",".-..","--","-.","---",".--.","--.-",".-.","...","-","..-","...-",".--","-..-","-.--","--..";
std::string reverseMorseAlphabet[27] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', '0', '1', '2', '3', '4', '5', '6', '7', '8', '9'}; // 字母与摩尔斯对应
// 转换函数
std::string encodeToMorse(const std::string &word) {
std::string result;
for (char c : word) {
if (isalpha(c)) {
result += morseAlphabet[c - 'A'];
} else if (isdigit(c)) {
result += morseAlphabet[c - '0' + 26]; // 将数字转为小写字母再编码
}
}
return result;
}
std::string decodeFromMorse(const std::string &morse) {
std::string decodedWord;
std::istringstream iss(morse);
char currentChar;
while (iss >> currentChar) {
decodedWord += reverseMorseAlphabet[currentChar];
}
return decodedWord;
}
int main() {
std::string input;
std::cout << "Enter text to convert to Morse or Morse code to decode: ";
std::getline(std::cin, input);
if (input.find_first_of(".-") != std::string::npos) { // 判断是否为摩尔斯电码
std::cout << "Decoded Text: " << decodeFromMorse(input) << "\n";
} else {
std::cout << "Encoded Morse Code: " << encodeToMorse(input) << "\n";
}
return 0;
}
```
阅读全文
相关推荐
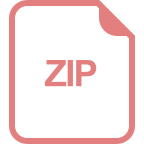
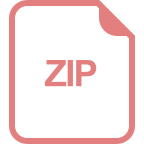
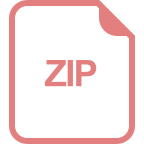
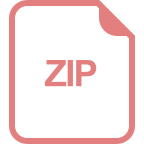
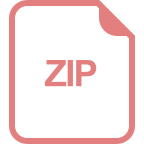
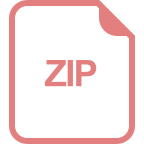
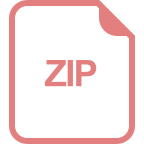
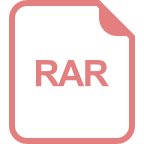
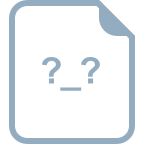
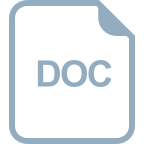
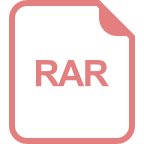
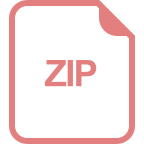
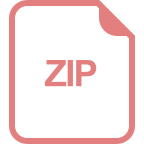
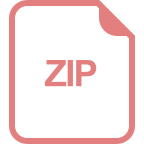
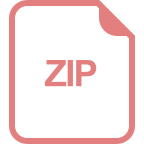