C# string.TrimEnd 与 string.remove
时间: 2024-09-13 20:11:16 浏览: 42
`string.TrimEnd()` 和 `string.Remove()` 都是 .NET 中用于处理字符串的方法,不过它们的功能略有不同。
`string.TrimEnd()` 方法的作用是从字符串的结尾处删除指定字符集(默认是空格、制表符、换页符、换行符、回车符和引号)的所有字符,直到字符串结束或遇到非指定字符为止。这个方法不会改变原始字符串,而是返回一个新的字符串。
例如:
```csharp
string original = "Hello, world! ";
string trimmed = original.TrimEnd(); // 输出 "Hello, world!"
```
而 `string.Remove()` 方法则是直接从原字符串中移除指定位置范围内的字符。它需要两个参数,第一个参数是要开始移除的位置索引(包括该位置),第二个参数是移除的长度。如果省略长度,则会移除从给定索引到字符串结束的所有字符。
例如:
```csharp
string original = "Hello, world!";
int startIndex = 7;
int length = 5; // 移除 "world"
string removed = original.Remove(startIndex, length); // 输出 "Hell,"
```
相关问题
c#中字符串函数大全
在C#中,字符串是一种特殊的数据类型,其内置了许多方便处理文本的函数。以下是一些常用的字符串函数示例:
1. `string.Length` 或 `.Length`: 返回字符串的字符数。
```csharp
string str = "Hello";
int length = str.Length; // length will be 5
```
2. `string.IsNullOrEmpty()` 或 `.IsNullOrWhiteSpace()`: 检查字符串是否为空或只包含空格。
```csharp
string str;
if (string.IsNullOrEmpty(str)) {
Console.WriteLine("String is null or empty");
}
```
3. `string.Concat()`: 连接两个或更多字符串。
```csharp
string combinedStr = string.Concat("Hello", " ", "World"); // combinedStr will be "Hello World"
```
4. `string.Substring()` 或 `string.Slice()`: 提取子串。
```csharp
string str = "HelloWorld";
string subStr = str.Substring(6); // subStr will be "World"
```
5. `string.IndexOf()` 或 `IndexOf(string, int)`: 查找指定字符或子串的位置。
```csharp
int index = str.IndexOf('W'); // index will be 6
```
6. `string.Replace()` 或 `Replace(oldValue, newValue)`: 替换字符串中的某个部分。
```csharp
string newStr = str.Replace("World", "Universe"); // newStr will be "Hello Universe"
```
7. `string.Split()` 或 `Split(char[])`: 将字符串分割成子字符串数组。
```csharp
string[] words = str.Split(' '); // words will contain ["Hello", "World"]
```
8. `string.Trim()` 或 `TrimStart()`、`TrimEnd()`: 移除字符串两端的空白字符。
```csharp
string trimmedStr = str.Trim(); // trimmedStr will remove leading and trailing spaces
```
还有很多其他函数,如格式化字符串(`string.Format()`)、正则表达式匹配(`Regex*`类)等。
阅读全文
相关推荐



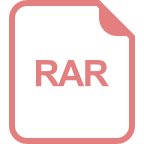



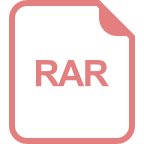

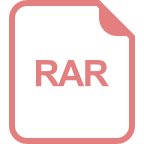





