c++如何在子线程中使用控件变量
时间: 2024-04-19 10:28:07 浏览: 13
在C++中使用控件变量的一般方法是将控件的操作限制在主线程中,因为大多数图形用户界面(GUI)框架都是单线程的。这意味着在主线程之外直接操作控件可能会导致不可预料的行为。
如果你想在子线程中使用控件变量,一种常见的方法是使用消息队列或事件队列来实现线程间通信。以下是一个基本的示例:
1. 在主线程中,创建一个消息队列或事件队列,用于子线程向主线程发送消息。
2. 在子线程中,通过将消息发送到队列来请求对控件的操作。
3. 主线程在接收到消息后,使用控件变量执行相应的操作。
下面是一个示例代码,演示了如何在子线程中更新一个文本标签控件:
```cpp
#include <iostream>
#include <thread>
#include <queue>
// 向主线程发送的消息结构体
struct ThreadMessage {
std::string text;
};
// 模拟一个文本标签控件
class TextLabel {
public:
void setText(const std::string& text) {
// 更新文本标签的操作
std::cout << "Updating text label: " << text << std::endl;
}
};
// 主线程函数
void mainThreadFunc(std::queue<ThreadMessage>& msgQueue, TextLabel& label) {
while (true) {
// 从队列中获取消息
if (!msgQueue.empty()) {
ThreadMessage msg = msgQueue.front();
msgQueue.pop();
// 更新控件变量
label.setText(msg.text);
}
}
}
// 子线程函数
void childThreadFunc(std::queue<ThreadMessage>& msgQueue) {
// 模拟子线程发送消息给主线程
ThreadMessage msg;
msg.text = "Hello from child thread!";
msgQueue.push(msg);
}
int main() {
std::queue<ThreadMessage> msgQueue;
TextLabel label;
// 创建主线程
std::thread mainThread(mainThreadFunc, std::ref(msgQueue), std::ref(label));
// 创建子线程
std::thread childThread(childThreadFunc, std::ref(msgQueue));
// 等待线程完成
childThread.join();
mainThread.join();
return 0;
}
```
在此示例中,子线程通过向消息队列中添加消息来请求对控件的操作。主线程则负责从队列中获取消息,并使用控件变量执行相应的操作。请注意,此示例只是一个基本的演示,实际中的实现可能因框架和需求而有所不同。
相关推荐
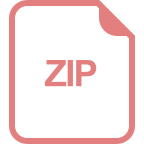
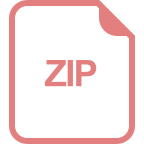
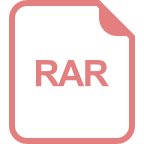














