多线程用threadlocal和不用threadlocal的java代码演示区别
时间: 2024-06-04 12:04:44 浏览: 16
当我们在Java中使用多线程时,有时候我们需要为每个线程创建一个独立的变量,这个时候我们可以使用ThreadLocal类来实现。
下面是一个使用ThreadLocal的Java代码示例:
```
public class ThreadLocalDemo {
private static final ThreadLocal<String> threadLocal = new ThreadLocal<>();
public static void main(String[] args) throws InterruptedException {
Thread thread1 = new Thread(() -> {
threadLocal.set("Thread1 local variable");
System.out.println(threadLocal.get());
threadLocal.remove();
});
Thread thread2 = new Thread(() -> {
threadLocal.set("Thread2 local variable");
System.out.println(threadLocal.get());
threadLocal.remove();
});
thread1.start();
thread2.start();
thread1.join();
thread2.join();
}
}
```
上面的代码中,我们创建了两个线程,每个线程都设置了自己的局部变量,并在控制台上打印出来。由于ThreadLocal类为每个线程提供了独立的实例,因此两个线程的局部变量不会互相干扰。
下面是一个不使用ThreadLocal的Java代码示例:
```
public class NoThreadLocalDemo {
private static String localVariable;
public static void main(String[] args) throws InterruptedException {
Thread thread1 = new Thread(() -> {
localVariable = "Thread1 local variable";
System.out.println(localVariable);
});
Thread thread2 = new Thread(() -> {
localVariable = "Thread2 local variable";
System.out.println(localVariable);
});
thread1.start();
thread2.start();
thread1.join();
thread2.join();
}
}
```
上面的代码中,我们同样创建了两个线程,并且在每个线程中设置了局部变量。但是由于局部变量是静态的,因此两个线程的局部变量会互相干扰,导致输出结果并不是我们期望的。
相关推荐
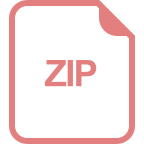
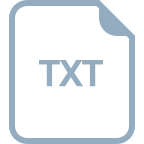
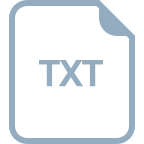
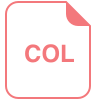
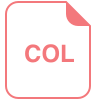
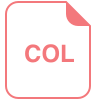
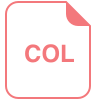
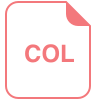









