如何在richtextbox中已有的特定字符位置插入一个空格?
时间: 2024-10-25 15:14:13 浏览: 36
在Windows Forms的RichTextBox中,要在已有特定字符的位置插入一个空格,你可以先找到该位置,然后使用`InsertCharacter`方法插入空格。下面是一个简单的步骤说明:
1. 获取光标当前的位置(索引),例如假设你想在单词“hello”中的第一个字母“h”之后插入空格,你需要计算出"hello"的长度(包括空格):
```csharp
int currentPosition = richTextBox.SelectionStart; // 当前光标位置
int positionToInsertSpace = currentPosition + "hello".Length; // 计算插入位置
```
2. 确保位置在有效范围内,避免超出文本长度:
```csharp
if (positionToInsertSpace <= richTextBox.TextLength) {
// 插入空格
richTextBox.InsertCharacter(positionToInsertSpace, ' ');
}
```
3. 选择插入后的字符以便显示:
```csharp
richtTextBox.Select(positionToInsertSpace, 1);
```
如果要在WPF的RichTextBox中做同样的事情,可以使用`TextRange`和`Insert`方法,过程大致相似。
记得在实际操作前,先备份或确认你不会意外覆盖其他内容,特别是处理用户输入的时候。
相关问题
在保证richTextBox里面内容不变的前提下,如何在richtextbox中已有字符中间插入一个空格
要在RichTextBox中已有字符中间插入一个空格而不改变原有的内容,可以先获取插入点的位置(即光标所在位置),然后执行插入操作。以下是步骤:
1. 获取插入点:你可以使用`GetSelectionStart()`和`GetSelectionLength()`方法来分别获得插入点的位置和选区长度。如果没有选区,则只有一行文本,可以用`GetTextRange()`方法从当前位置开始获取文本。
```csharp
int insertPoint = richTextBox.SelectionStart;
if (insertPoint == richTextBox.Text.Length)
{
// 如果没有选区,假设在行尾
insertPoint = richTextBox.GetLineFromCharIndex(richTextBox.Text.Length);
}
```
2. 插入空格:创建一个新的`RTFCharacterRun`对象,并设置其文本为空格,然后用`InsertRunAt()`方法在指定位置插入这个运行。
```csharp
string space = " ";
RTFCharacterRun run = new RTFCharacterRun(space);
richTextBox.InsertRun(insertPoint, run);
```
这会在当前插入点处插入一个空格。注意,如果你需要插入多个空格,可以在创建`RTFCharacterRun`时将`space`变量设为所需的空格数。
在richTextBox已有内容的指定位置插入一个空格如何实现
在.NET的RichTextBox控件中,要在已有的文本指定位置插入一个空格,你需要获取该位置的索引,然后调用`InsertString`方法。以下是步骤:
1. 首先,确定你要插入空格的位置。假设你已经有了当前的光标位置或字符索引。
```csharp
int positionToInsert = richTextBox.SelectionStart; // 获取光标位置
```
2. 然后,使用`InsertString`方法在指定位置插入空格。
```csharp
richtTextBox.SelectedText = richTextBox.SelectedText.Insert(positionToInsert, " "); // 在位置后插入空格
```
3. 如果你想保持光标在插入点,调用`MoveSelection`方法:
```csharp
richtTextBox.MoveSelection(Cursor.Left); // 将光标移动到插入点左边,表示空格已经插入
```
完整示例:
```csharp
if (richtTextBox.SelectedText.Length > 0) {
int positionToInsert = richTextBox.SelectionStart;
richtTextBox.SelectedText = richTextBox.SelectedText.Insert(positionToInsert, " ");
richTextBox.MoveSelection(Cursor.Left);
}
```
这样就实现了在RichTextBox已有内容的指定位置插入了一个空格。
阅读全文
相关推荐
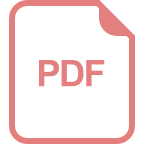
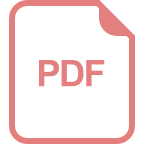
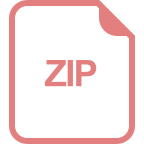












