c++停车场管理系统
时间: 2023-12-22 22:27:52 浏览: 52
根据提供的引用内容,这个停车场管理系统是一个纯文件操作的c++程序,没有使用链表。它可以在Dev5.10c++中打开。具体实现的功能包括车辆进入停车场、车辆离开停车场、显示停车场内车辆信息等。下面是一个简单的c++停车场管理系统的实现:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std;
// 定义车辆结构体
struct Car {
string license; // 车牌号
string brand; // 车品牌
string color; // 车颜色
};
// 定义停车场类
class ParkingLot {
public:
ParkingLot() {
// 从文件中读取停车场信息
ifstream infile("parking_lot.txt");
if (infile.is_open()) {
string line;
while (getline(infile, line)) {
Car car;
car.license = line.substr(0, 7);
car.brand = line.substr(8, 10);
car.color = line.substr(19, 5);
cars.push_back(car);
}
infile.close();
}
}
// 车辆进入停车场
void enter(Car car) {
if (cars.size() < capacity) {
cars.push_back(car);
saveToFile();
cout << "车辆进入停车场成功!" << endl;
} else {
cout << "停车场已满,无法进入!" << endl;
}
}
// 车辆离开停车场
void leave(string license) {
for (int i = 0; i < cars.size(); i++) {
if (cars[i].license == license) {
cars.erase(cars.begin() + i);
saveToFile();
cout << "车辆离开停车场成功!" << endl;
return;
}
}
cout << "停车场中没有该车辆!" << endl;
}
// 显示停车场内车辆信息
void showCars() {
if (cars.size() == 0) {
cout << "停车场内没有车辆!" << endl;
} else {
cout << "停车场内车辆信息:" << endl;
for (int i = 0; i < cars.size(); i++) {
cout << "车牌号:" << cars[i].license << ",车品牌:" << cars[i].brand << ",车颜色:" << cars[i].color << endl;
}
}
}
private:
vector<Car> cars; // 停车场内车辆信息
int capacity = 10; // 停车场容量
// 将停车场信息保存到文件中
void saveToFile() {
ofstream outfile("parking_lot.txt");
if (outfile.is_open()) {
for (int i = 0; i < cars.size(); i++) {
outfile << cars[i].license << " " << cars[i].brand << " " << cars[i].color << endl;
}
outfile.close();
}
}
};
int main() {
ParkingLot parkingLot;
while (true) {
cout << "请选择操作:1.车辆进入停车场 2.车辆离开停车场 3.显示停车场内车辆信息 4.退出" << endl;
int choice;
cin >> choice;
if (choice == 1) {
Car car;
cout << "请输入车牌号:";
cin >> car.license;
cout << "请输入车品牌:";
cin >> car.brand;
cout << "请输入车颜色:";
cin >> car.color;
parkingLot.enter(car);
} else if (choice == 2) {
string license;
cout << "请输入车牌号:";
cin >> license;
parkingLot.leave(license);
} else if (choice == 3) {
parkingLot.showCars();
} else if (choice == 4) {
break;
} else {
cout << "输入有误,请重新输入!" << endl;
}
}
return 0;
}
```
相关推荐
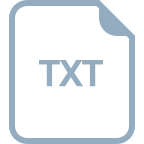














