编写一个函数,删除列表中指定元素。要求列表和指定删除的元素都为参数。python
时间: 2024-12-17 13:40:02 浏览: 6
在Python中,你可以编写一个名为`remove_element`的函数,该函数接受一个列表和一个要删除的元素作为参数,然后遍历列表并移除指定的元素。以下是这个函数的一个简单实现:
```python
def remove_element(lst, element):
try:
lst.remove(element) # 尝试从列表中移除指定元素
except ValueError: # 如果元素不在列表中,ValueError会被抛出
print(f"{element} not found in the list.")
return lst
# 使用示例
numbers = [1, 2, 3, 4, 5]
element_to_remove = 3
new_list = remove_element(numbers, element_to_remove)
print(f"The updated list is: {new_list}")
```
此函数首先尝试通过`remove()`方法从列表中移除指定元素,如果元素不存在则捕获`ValueError`异常并打印一条消息。最后返回修改后的列表。
相关问题
3. 编写程序从一给定的顺序表A中删除值在x,y(x<=y)之间的所有元素。要求:写出实现删除的子函数并记录运行结果。 提示: 1)方法:逐一比较每个元素的值,若元素值在X,Y之间,则删除。
为了编写这个程序,我们可以创建一个名为`delete_elements`的子函数,该函数接受一个顺序表A和两个整数x和y作为参数。顺序列表通常表示为数组或者链表的形式。以下是使用链表为例的伪代码实现:
```python
class Node:
def __init__(self, value):
self.value = value
self.next = None
def delete_elements(A, x, y):
# 定义头节点
head = A[0] if A else None
# 遍历链表
current = head
prev = None
while current is not None:
if current.value >= x and current.value <= y:
# 如果当前节点的值在范围内,删除它
if prev is None:
head = current.next
else:
prev.next = current.next
else:
prev = current
current = current.next
return head # 返回修改后的链表头部
# 示例
# 初始化一个顺序列表
A = [1, 2, 3, 4, 5, 6, 7]
x = 3
y = 6
# 删除指定范围内的元素
new_list = delete_elements(A, x, y)
# 打印处理后的列表
while new_list is not None:
print(new_list.value)
new_list = new_list.next
阅读全文
相关推荐












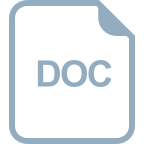
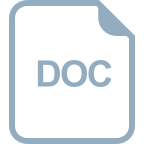



