C#怎么批量读写INI
时间: 2023-10-19 11:55:30 浏览: 76
在C#中,你可以使用 `System.IO` 命名空间中的 `StreamReader` 和 `StreamWriter` 类来实现批量读写INI文件。以下是一个简单的示例代码:
```csharp
using System.IO;
// 批量读取INI文件
public static Dictionary<string, string> ReadIniFile(string filePath)
{
Dictionary<string, string> iniData = new Dictionary<string, string>();
using (StreamReader reader = new StreamReader(filePath))
{
string line;
string currentSection = "";
while ((line = reader.ReadLine()) != null)
{
line = line.Trim();
if (line.StartsWith("[") && line.EndsWith("]"))
{
currentSection = line.Substring(1, line.Length - 2);
}
else if (!string.IsNullOrWhiteSpace(line) && !line.StartsWith(";"))
{
int separatorIndex = line.IndexOf('=');
if (separatorIndex > 0)
{
string key = line.Substring(0, separatorIndex).Trim();
string value = line.Substring(separatorIndex + 1).Trim();
if (!string.IsNullOrEmpty(currentSection))
{
key = currentSection + ":" + key;
}
iniData[key] = value;
}
}
}
}
return iniData;
}
// 批量写入INI文件
public static void WriteIniFile(string filePath, Dictionary<string, string> iniData)
{
using (StreamWriter writer = new StreamWriter(filePath))
{
foreach (var item in iniData)
{
string[] sectionsAndKey = item.Key.Split(':');
string section = sectionsAndKey.Length > 1 ? sectionsAndKey[0] : "";
string key = sectionsAndKey.Length > 1 ? sectionsAndKey[1] : sectionsAndKey[0];
string value = item.Value;
if (!string.IsNullOrEmpty(section))
{
writer.WriteLine($"[{section}]");
}
writer.WriteLine($"{key}={value}");
}
}
}
```
使用示例:
```csharp
// 批量读取INI文件
string filePath = "path/to/your/inifile.ini";
Dictionary<string, string> iniData = ReadIniFile(filePath);
// 修改或添加INI配置项
iniData["Section1:Key1"] = "Value1";
iniData["Section2:Key2"] = "Value2";
// 批量写入INI文件
WriteIniFile(filePath, iniData);
```
请确保你已包含 `System.IO` 命名空间,并将 `path/to/your/inifile.ini` 替换为你的实际INI文件路径。
相关推荐
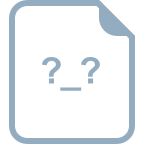
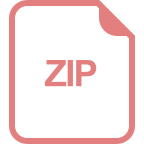














