easyexcel从数据库导出excel
时间: 2023-08-17 10:07:16 浏览: 74
您可以使用 EasyExcel 库来从数据库导出 Excel 文件。下面是一个示例代码,展示了如何使用 EasyExcel 和 JDBC 连接数据库,并将查询结果导出到 Excel 文件中:
```java
import com.alibaba.excel.EasyExcel;
import com.alibaba.excel.metadata.Sheet;
import com.alibaba.excel.metadata.TableStyle;
import com.alibaba.excel.support.ExcelTypeEnum;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class DatabaseToExcelExporter {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/database_name";
String username = "username";
String password = "password";
try {
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
Statement statement = connection.createStatement();
String sql = "SELECT * FROM table_name";
ResultSet resultSet = statement.executeQuery(sql);
// 创建 Excel 文件
String outputPath = "output.xlsx";
EasyExcel.write(outputPath)
.excelType(ExcelTypeEnum.XLSX)
.registerWriteHandler(getTableStyle()) // 设置表格样式(可选)
.sheet("Sheet1")
.doWrite(resultSetToData(resultSet));
resultSet.close();
statement.close();
connection.close();
System.out.println("导出成功!");
} catch (Exception e) {
e.printStackTrace();
}
}
// 将 ResultSet 转换为二维数组,用于 EasyExcel 导出
private static Object[][] resultSetToData(ResultSet resultSet) throws Exception {
int columnCount = resultSet.getMetaData().getColumnCount();
Object[][] data = new Object[columnCount][columnCount];
int rowIndex = 0;
while (resultSet.next()) {
Object[] row = new Object[columnCount];
for (int i = 0; i < columnCount; i++) {
row[i] = resultSet.getObject(i + 1);
}
data[rowIndex++] = row;
}
return data;
}
// 设置表格样式(可选)
private static TableStyle getTableStyle() {
TableStyle tableStyle = new TableStyle();
// 设置表头样式
tableStyle.setTableHeadBackGroundColor(IndexedColors.GREY_25_PERCENT.getIndex());
// 设置内容行样式
tableStyle.setTableContentBackGroundColor(IndexedColors.WHITE.getIndex());
return tableStyle;
}
}
```
请根据实际情况修改 `url`、`username`、`password`、`outputPath`、`sql`、`table_name` 和 `database_name` 等参数。这段代码会将查询结果导出到名为 "output.xlsx" 的 Excel 文件中。您还可以使用 `getTableStyle()` 方法自定义表格样式。
注意:在使用该代码前,请确保已添加 EasyExcel 和 JDBC 的依赖。
相关推荐
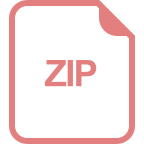














