c++ 删除文件示例
时间: 2023-11-24 14:45:42 浏览: 70
你可以使用 C 语言中的 remove() 函数来删除文件。remove() 函数的原型如下:
```c
int remove(const char *filename);
```
该函数接受一个字符串参数作为文件名,并返回一个整数值来表示操作是否成功。如果文件删除成功,则返回 0;如果删除失败,则返回 -1。
以下是一个示例程序,展示了如何使用 remove() 函数删除文件:
```c
#include <stdio.h>
int main() {
char filename[100];
printf("请输入要删除的文件名:");
scanf("%s", filename);
int result = remove(filename);
if(result == 0) {
printf("文件删除成功!\n");
} else {
printf("文件删除失败。\n");
}
return 0;
}
```
请注意,使用 remove() 函数删除文件时要小心,因为被删除的文件无法恢复。确保你真正想要删除的是该文件,以免误操作。
相关问题
qt文件拷贝c++示例
以下是一个使用Qt的C++示例,演示如何将一个文件从一个位置复制到另一个位置:
```c++
#include <QFile>
#include <QDir>
bool copyFile(const QString& sourceFile, const QString& destinationDir)
{
// 获取源文件名和目标文件名
QFileInfo fileInfo(sourceFile);
QString destinationFile = destinationDir + QDir::separator() + fileInfo.fileName();
// 如果目标文件已经存在,则删除它
if (QFile::exists(destinationFile))
QFile::remove(destinationFile);
// 尝试复制文件
if (!QFile::copy(sourceFile, destinationFile))
return false;
return true;
}
```
使用该函数时,只需提供源文件的完整路径和目标目录的完整路径即可。例如:
```c++
QString sourceFile = "/path/to/source/file.txt";
QString destinationDir = "/path/to/destination/directory";
if (copyFile(sourceFile, destinationDir)) {
// 文件已成功复制
} else {
// 文件复制失败
}
```
C++删除文件
在C++中,可以使用remove函数来删除文件。该函数的原型如下:
```
int remove(const char* filename);
```
其中,filename参数是要删除的文件名。
示例代码如下:
```c++
#include <iostream>
#include <cstdio>
using namespace std;
int main()
{
const char* filename = "test.txt";
int ret = remove(filename);
if (ret == 0)
cout << "文件删除成功!" << endl;
else
cout << "文件删除失败!" << endl;
return 0;
}
```
需要注意的是,remove函数只能删除当前工作目录下的文件,不能删除其他目录下的文件,也不能删除只读文件。如果文件被打开或者被占用,删除操作也会失败。
相关推荐
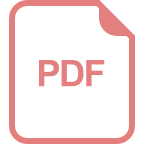
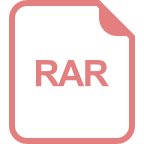
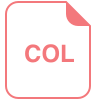
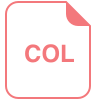
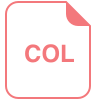









