Unity2D利用代码实现鱼的集群跟随
时间: 2024-04-12 09:32:22 浏览: 19
要实现鱼的集群跟随效果,你可以使用一种称为"Boids算法"的群体行为算法。Boids算法包括三个主要的行为:对齐、凝聚和分离。下面是一个示例代码,演示了如何实现鱼的集群跟随效果:
```C#
using UnityEngine;
public class Fish : MonoBehaviour
{
public float speed = 5f; // 鱼的移动速度
public float neighborRadius = 3f; // 邻居半径
public float alignmentWeight = 1f; // 对齐权重
public float cohesionWeight = 1f; // 凝聚权重
public float separationWeight = 1f; // 分离权重
private Vector2 direction; // 鱼的移动方向
private void Start()
{
direction = Random.insideUnitCircle.normalized;
}
private void Update()
{
Vector2 alignment = Vector2.zero;
Vector2 cohesion = Vector2.zero;
Vector2 separation = Vector2.zero;
int neighborCount = 0;
Collider2D[] colliders = Physics2D.OverlapCircleAll(transform.position, neighborRadius);
foreach (var collider in colliders)
{
if (collider.gameObject != gameObject) // 排除自身
{
Fish otherFish = collider.GetComponent<Fish>();
if (otherFish != null)
{
// 对齐
alignment += otherFish.transform.up;
// 凝聚
cohesion += otherFish.transform.position;
// 分离
separation += (Vector2)(transform.position - otherFish.transform.position);
neighborCount++;
}
}
}
if (neighborCount > 0)
{
// 平均对齐
alignment /= neighborCount;
// 平均凝聚
cohesion /= neighborCount;
// 计算最终的移动方向
Vector2 desiredDirection = (alignment * alignmentWeight + cohesion * cohesionWeight + separation * separationWeight).normalized;
direction = Vector2.Lerp(direction, desiredDirection, Time.deltaTime);
}
// 更新鱼的位置
transform.position += (Vector3)(direction * speed * Time.deltaTime);
// 让鱼的头部朝向移动方向
transform.up = direction;
}
}
```
在这个示例代码中,每条鱼都会检测周围的邻居,并根据邻居的位置和朝向进行对齐、凝聚和分离操作。然后通过权重调整这些行为的影响力,并计算出最终的移动方向。请将此脚本附加到鱼的游戏对象上,并根据你的需求调整参数,如速度、邻居半径和权重等。这样,鱼就会在集群中跟随并与邻居保持一定的对齐、凝聚和分离关系。
相关推荐
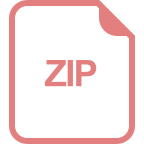









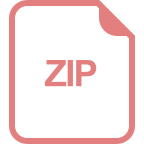
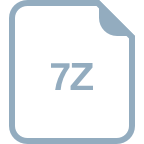