一个完整的用C++编写的程序,它会接受一个浮点数(double 类型)的输入,并找出该浮点数中整数部分和小数部分的最大及最小数字。完整的程序
时间: 2024-09-16 12:04:38 浏览: 45
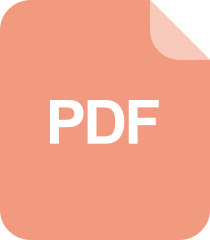
基于C++浮点数(float、double)类型数据比较与转换的详解
当然可以。下面是一个简单的C++程序,用于接收用户输入的浮点数,将其拆分为整数部分和小数部分,然后分别找到最大和最小的数字:
```cpp
#include <iostream>
#include <cmath>
// Function to extract integer and decimal parts of a float
void splitAndFindMinMax(double number) {
int intPart = static_cast<int>(number); // Integer part (truncating)
double fracPart = std::modf(number, &intPart); // Decimal part (remaining fraction)
// Finding max and min of the integer part
int minInt = intPart;
int maxInt = intPart;
// If there is a fractional part, find its minimum and maximum
if (fracPart != 0.0) {
minInt = std::min(minInt, static_cast<int>(fracPart));
maxInt = std::max(maxInt, static_cast<int>(fracPart));
}
std::cout << "Integer Part Min: " << minInt << "\n";
std::cout << "Integer Part Max: " << maxInt << "\n";
// Since the decimal part is always between 0 and 1, we can directly print it as is
std::cout << "Decimal Part: " << fracPart << "\n";
}
int main() {
double inputNumber;
std::cout << "Enter a floating point number: ";
std::cin >> inputNumber;
// Call the function with user input
splitAndFindMinMax(inputNumber);
return 0;
}
```
当你运行这个程序并输入一个浮点数时,它将分别显示整数部分的最小值、最大值以及小数部分。
阅读全文
相关推荐
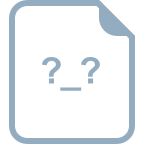
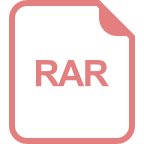















