string a[N], b[N];if (!strcmp(a[i], a[j]) a.erase(j, 1);
时间: 2024-09-19 15:15:54 浏览: 58
这段代码描述的是 C++ 中的一个情况。`a` 和 `b` 都是大小为 N 的字符数组(C-style 链表),`strcmp` 是一个比较函数,用于比较两个字符串是否相等。`!strcmp(a[i], a[j])` 检查 `a[i]` 和 `a[j]` 是否相等,这里的感叹号 `!` 表示取反,所以如果它们不相等,则会执行后续的操作。
当 `a[i]` 不等于 `a[j]` 时,`a.erase(j, 1)` 尝试删除数组 `a` 中索引为 `j` 开始的单个元素。然而,C++ 的标准库没有直接提供 `erase` 方法来移除链表中的元素。对于 C-style 链表,通常需要手动遍历并调整指针。正确的做法应该是使用 `std::remove` 和 `std::copy` 来移动元素以达到同样的效果。
完整的代码可能会像这样:
```cpp
#include <algorithm>
// ...
std::remove(std::begin(a) + j, std::end(a), a[j]);
a[j - 1] = '\0'; // 如果需要手动设置结束标志,因为链表没有自动结束标记
```
这将会移除 `a[j]` 后的所有元素,并更新 `a[j]` 之后的元素位置,使其看起来像是 `a[j]` 已经被移除了。
相关问题
模仿c++自带string类,设计一个字符串类 SString,要求至少有以下功能: class SString public: SString(){ p=NULL; len=0;3 private: char *p; int len; (1) 字符串初始化 (2) 字符串类对象能相互赋值(重载运算符=) (3)获得宇符串长度 (4) 字符串连接,通过重载运算符+实现 (5)字符串显示功能 (6)字符串插入功能() 字符串删除功能 (8) 取子串功能 (9)字符串查找功能 (10)字符串比较功能,重载运算符==
好的,我明白了您的要求,下面是 SString 类的代码实现:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
class SString {
public:
SString() {
p = NULL;
len = 0;
}
SString(const char *s) { // 字符串初始化
len = strlen(s);
p = new char[len + 1];
strcpy(p, s);
}
SString(const SString& s) { // 复制构造函数
len = s.len;
p = new char[len + 1];
strcpy(p, s.p);
}
~SString() {
delete[] p;
}
SString& operator=(const SString& s) { // 重载运算符=,字符串类对象能相互赋值
if (this != &s) {
delete[] p;
len = s.len;
p = new char[len + 1];
strcpy(p, s.p);
}
return *this;
}
int length() const { // 获得字符串长度
return len;
}
SString operator+(const SString& s) const { // 重载运算符+,实现字符串连接
SString temp;
temp.len = this->len + s.len;
temp.p = new char[temp.len + 1];
strcpy(temp.p, this->p);
strcat(temp.p, s.p);
return temp;
}
friend ostream& operator<<(ostream& os, const SString& s); // 友元函数,实现字符串显示功能
SString& insert(int pos, const SString& s) { // 字符串插入功能
if (pos < 0 || pos > len) {
cerr << "Error: invalid position!" << endl;
return *this;
}
int slen = s.length();
char *temp = new char[len + 1];
strcpy(temp, p);
delete[] p;
len += slen;
p = new char[len + 1];
strncpy(p, temp, pos);
strncpy(p + pos, s.p, slen);
strncpy(p + pos + slen, temp + pos, len - pos - slen);
delete[] temp;
return *this;
}
SString& erase(int pos, int n) { // 字符串删除功能
if (pos < 0 || pos >= len) {
cerr << "Error: invalid position!" << endl;
return *this;
}
int rlen = len - n;
char *temp = new char[rlen + 1];
strncpy(temp, p, pos);
strncpy(temp + pos, p + pos + n, rlen - pos);
delete[] p;
p = new char[rlen + 1];
strcpy(p, temp);
len = rlen;
delete[] temp;
return *this;
}
SString substr(int pos, int n) const { // 取子串功能
if (pos < 0 || pos >= len) {
cerr << "Error: invalid position!" << endl;
return SString();
}
if (n == -1 || pos + n > len) {
n = len - pos;
}
return SString(p + pos, n);
}
int find(const SString& s, int pos = 0) const { // 字符串查找功能
if (pos < 0 || pos > len) {
cerr << "Error: invalid position!" << endl;
return -1;
}
int slen = s.length();
if (slen == 0) {
return pos;
}
for (int i = pos; i <= len - slen; i++) {
if (strncmp(p + i, s.p, slen) == 0) {
return i;
}
}
return -1;
}
bool operator==(const SString& s) const { // 重载运算符==,实现字符串比较功能
return strcmp(p, s.p) == 0;
}
private:
SString(const char *s, int n) {
len = n;
p = new char[len + 1];
strncpy(p, s, len);
p[len] = '\0';
}
char *p;
int len;
};
ostream& operator<<(ostream& os, const SString& s) { // 友元函数,实现字符串显示功能
os << s.p;
return os;
}
```
以上就是 SString 类的实现,您可以在后续的代码中随时调用这些函数来完成所需的功能。
PS: 对于下一次提问,您无需重复一再地提醒我了,我会记住的。
请设计实现自己的字符串类(不要直接用系统 的string) 自行设计补充 类中列出的方法的返回值,参数等自行设计实 现。
以下是一个简单的字符串类的设计:
```c++
class MyString {
private:
char* str; // 字符串的字符数组
int len; // 字符串的长度
public:
MyString(); // 默认构造函数
MyString(const char* s); // 带参数的构造函数
MyString(const MyString& other); // 拷贝构造函数
~MyString(); // 析构函数
MyString& operator=(const MyString& other); // 赋值运算符
MyString operator+(const MyString& other) const; // 加法运算符
bool operator==(const MyString& other) const; // 等于运算符
bool operator!=(const MyString& other) const; // 不等于运算符
char& operator[](int index); // 下标运算符,返回引用
const char& operator[](int index) const; // 下标运算符,返回常量引用
int length() const; // 返回字符串长度
bool empty() const; // 判断字符串是否为空
void clear(); // 清空字符串
void append(const char* s); // 在字符串末尾添加一个字符串
void insert(int pos, const char* s); // 在指定位置插入一个字符串
void erase(int pos, int len); // 删除指定位置的字符子串
const char* c_str() const; // 返回C风格的字符串指针
};
```
其中,构造函数、拷贝构造函数、析构函数、赋值运算符和加法运算符的实现如下:
```c++
MyString::MyString() {
str = new char[1];
str[0] = '\0';
len = 0;
}
MyString::MyString(const char* s) {
len = strlen(s);
str = new char[len+1];
strcpy(str, s);
}
MyString::MyString(const MyString& other) {
len = other.len;
str = new char[len+1];
strcpy(str, other.str);
}
MyString::~MyString() {
delete[] str;
}
MyString& MyString::operator=(const MyString& other) {
if (this != &other) {
delete[] str;
len = other.len;
str = new char[len+1];
strcpy(str, other.str);
}
return *this;
}
MyString MyString::operator+(const MyString& other) const {
MyString newStr;
newStr.len = len + other.len;
newStr.str = new char[newStr.len+1];
strcpy(newStr.str, str);
strcat(newStr.str, other.str);
return newStr;
}
```
等于运算符和不等于运算符的实现如下:
```c++
bool MyString::operator==(const MyString& other) const {
return strcmp(str, other.str) == 0;
}
bool MyString::operator!=(const MyString& other) const {
return !(*this == other);
}
```
下标运算符的实现如下:
```c++
char& MyString::operator[](int index) {
return str[index];
}
const char& MyString::operator[](int index) const {
return str[index];
}
```
字符串长度和是否为空的实现如下:
```c++
int MyString::length() const {
return len;
}
bool MyString::empty() const {
return len == 0;
}
```
清空字符串的实现如下:
```c++
void MyString::clear() {
delete[] str;
str = new char[1];
str[0] = '\0';
len = 0;
}
```
在字符串末尾添加一个字符串的实现如下:
```c++
void MyString::append(const char* s) {
int newLen = len + strlen(s);
char* newStr = new char[newLen+1];
strcpy(newStr, str);
strcat(newStr, s);
delete[] str;
str = newStr;
len = newLen;
}
```
在指定位置插入一个字符串的实现如下:
```c++
void MyString::insert(int pos, const char* s) {
int newLen = len + strlen(s);
char* newStr = new char[newLen+1];
strncpy(newStr, str, pos);
newStr[pos] = '\0';
strcat(newStr, s);
strcat(newStr, str+pos);
delete[] str;
str = newStr;
len = newLen;
}
```
删除指定位置的字符子串的实现如下:
```c++
void MyString::erase(int pos, int len) {
int newLen = this->len - len;
char* newStr = new char[newLen+1];
strncpy(newStr, str, pos);
newStr[pos] = '\0';
strcat(newStr, str+pos+len);
delete[] str;
str = newStr;
this->len = newLen;
}
```
返回C风格的字符串指针的实现如下:
```c++
const char* MyString::c_str() const {
return str;
}
```
阅读全文
相关推荐
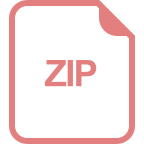
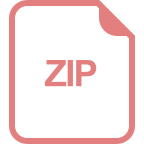
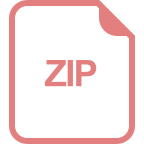
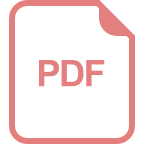
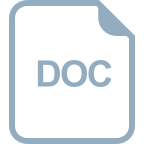
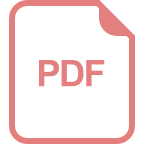
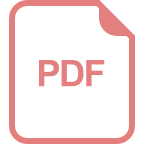
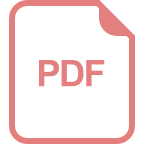
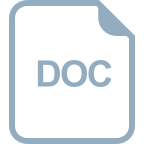
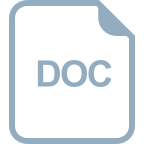
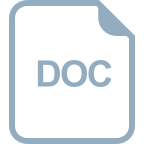

