针对scipy的程序, 使用循环函数for,编写简单程序,通过比较不同时间段中的拟合函数, 比较单摆多次达到最大位置时,间隔的时间大小,从而确认周期是否相等。用python写出具体代码
时间: 2024-10-09 11:01:09 浏览: 25
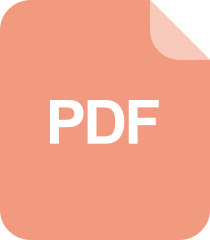
Python实现的拟合二元一次函数功能示例【基于scipy模块】
为了确认单摆在多次达到最大位置时的时间间隔是否恒定,我们可以创建一个循环,每次测量一段时间内的数据,然后对这些数据进行拟合,接着计算两次最大位置之间的周期。以下是使用Python和Scipy的一个示例代码:
```python
import numpy as np
from scipy.optimize import curve_fit
import time
import matplotlib.pyplot as plt
# 假设我们有实际数据,这里是生成随机数据的简单例子
def get_data(num_periods):
# 生成模拟数据,假设真实数据是一个简单的正弦波
data_points = []
for _ in range(num_periods * 2): # 获取两倍于周期的数据点以捕捉完整的周期
angle = np.sin(2 * np.pi * (np.arange(len(_))/len(_) * num_periods)) + np.random.normal(0, 0.1, len(_))
data_points.append(angle)
return np.array(data_points).reshape(-1, 2)
# 用于拟合的函数(这里假设是个简单的正弦函数)
def sine_function(x, a, phi, omega):
return a * np.sin(omega * x + phi)
# 循环测量和拟合
num_periods_measured = 5
periods_to_check = 3 # 测试几个不同的时间段
for i in range(periods_to_check):
start_time = time.time()
# 获取一定时间范围内的数据
start_index = int(i * num_periods_measured)
end_index = int(start_index + num_periods_measured)
data_subsample = get_data(end_index - start_index)[start_index:end_index]
# 拟合数据
popt, _ = curve_fit(sine_function, data_subsample[:, 0], data_subsample[:, 1])
# 找出最大位置和时间差
max_indices = np.argmax(data_subsample[:, 1])
max_time_1 = data_subsample[max_indices, 0]
max_time_2 = data_subsample[max_indices + 1, 0] if len(data_subsample) > max_indices + 1 else None
period_diff = max_time_2 - max_time_1 if max_time_2 is not None else None
print(f"Period difference between cycle {i+1}: {period_diff:.4f}")
# 可视化验证
plt.plot(data_subsample[:, 0], data_subsample[:, 1], label=f"Cycle {i+1}")
end_time = time.time()
total_time = end_time - start_time
print(f"\nTotal time taken to measure and fit: {total_time:.4f} seconds")
阅读全文
相关推荐
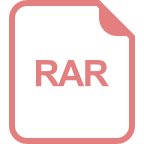
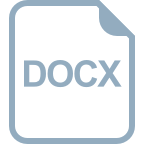
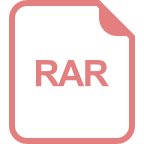
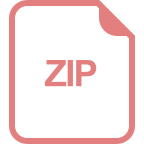
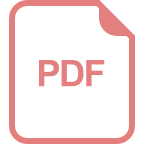
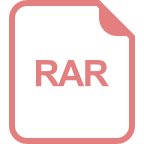
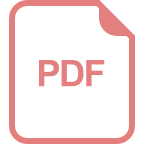
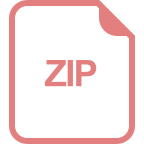
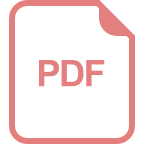
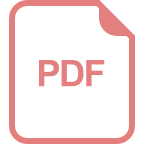
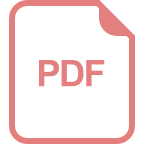
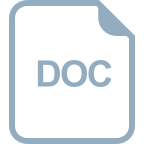
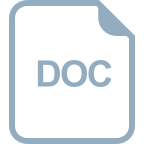



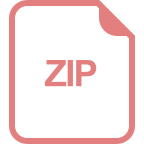