C# winfrom httpserver POST GET json 异步
时间: 2024-10-28 20:06:47 浏览: 33
在C# Windows Forms应用中,如果你想要处理HTTP请求(如POST、GET),并解析JSON数据,通常会使用`System.Net.Http`库,特别是配合异步操作,可以提高性能和用户体验。以下是使用`HttpClient`进行异步HTTP通信的一个简单示例:
首先,你需要添加对`System.Net.Http`的引用。
```csharp
using System;
using System.Net.Http;
using System.Threading.Tasks;
using Newtonsoft.Json; // 使用Json.NET库解析JSON
public partial class YourForm : Form
{
private HttpClient httpClient;
public YourForm()
{
InitializeComponent();
httpClient = new HttpClient();
}
private async Task SendPostRequestAsync(string url, object data)
{
try
{
HttpContent content = new StringContent(JsonConvert.SerializeObject(data), Encoding.UTF8, "application/json");
HttpResponseMessage response = await httpClient.PostAsync(url, content);
if (response.IsSuccessStatusCode)
{
string responseBody = await response.Content.ReadAsStringAsync();
dynamic result = JsonConvert.DeserializeObject(responseBody); // 解析响应的JSON
Console.WriteLine($"Response: {result}");
}
else
{
Console.WriteLine($"Error status code: {response.StatusCode}");
}
}
catch (Exception ex)
{
Console.WriteLine($"Error sending request: {ex.Message}");
}
}
// 示例按钮点击事件处理
private void buttonSend_Click(object sender, EventArgs e)
{
string postUrl = "http://your-api-url.com/endpoint";
// 假设data是一个包含你想发送的数据的对象
var data = new { key1 = "value1", key2 = "value2" };
SendPostRequestAsync(postUrl, data).Wait(); // 注意这里使用了同步等待,实际项目推荐使用async/await结构
}
}
```
在这个例子中,当用户点击`buttonSend`时,会发送一个POST请求到指定URL,并将JSON化的数据作为内容。然后,它会检查响应状态码并解析返回的JSON。
阅读全文
相关推荐
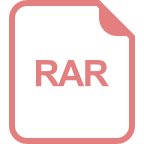
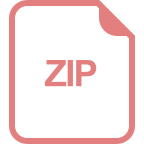

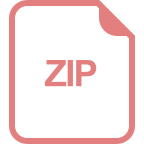
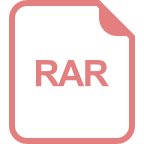
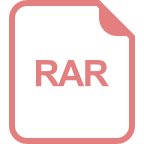
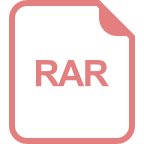
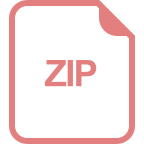
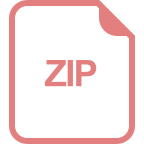
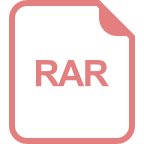
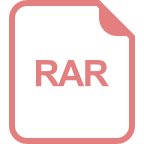
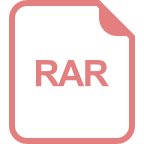
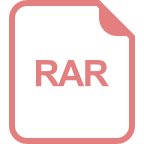
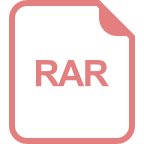
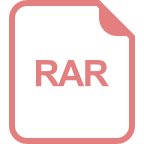
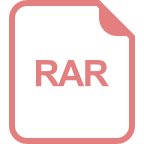
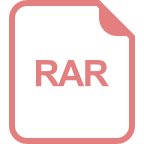
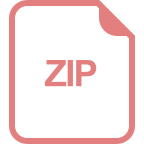
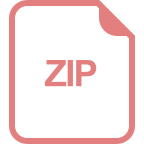