输入长度为n的一维整数数组各元素的值,按从小到大排列后输出.要求每行输出十个元素.
时间: 2023-09-10 07:01:59 浏览: 86
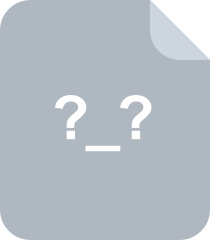
在键盘上输入一个3行3列矩阵的各个元素的值(值为整数),然后输出矩阵第一行与第三行元素之和,并在fun()函数中输出

这是一个简单的整数数组排序问题。可以使用排序算法进行解决,常见的有冒泡排序、插入排序、选择排序和快速排序等。
下面以快速排序算法为例,给出一个示例代码:
```java
public class ArraySorting {
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
quickSort(arr, low, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, high);
}
}
public static int partition(int[] arr, int low, int high) {
int pivot = arr[low];
while (low < high) {
while (low < high && arr[high] >= pivot) {
high--;
}
arr[low] = arr[high];
while (low < high && arr[low] <= pivot) {
low++;
}
arr[high] = arr[low];
}
arr[low] = pivot;
return low;
}
public static void printArray(int[] arr) {
for (int i = 0; i < arr.length; i++) {
System.out.print(arr[i] + " ");
if ((i + 1) % 10 == 0) { // 每行输出十个元素
System.out.println();
}
}
}
public static void main(String[] args) {
int[] arr = {3, 1, 5, 2, 4}; // 这里示意输入一个长度为5的整数数组
quickSort(arr, 0, arr.length - 1);
printArray(arr);
}
}
```
这个示例中,通过`quickSort`方法进行快速排序,再通过`printArray`方法按要求每行输出十个元素。
为了简化问题,这里示意输入了一个长度为5的整数数组,可以根据实际情况输入更多或少的元素,然后根据需要调整代码中的数组长度。
阅读全文
相关推荐
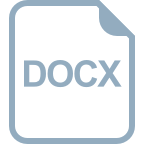

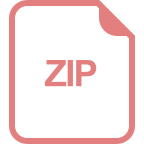
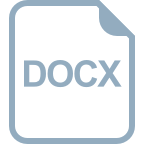
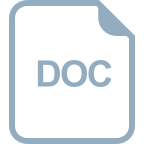
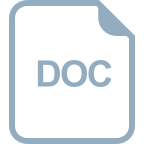
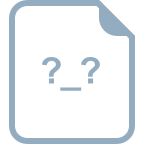
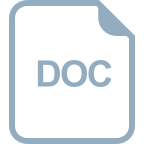
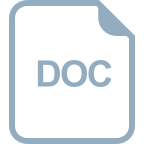
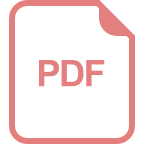
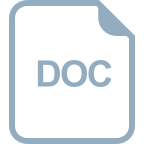



