如何在C语言中实现文件I/O操作来保存账户信息?
时间: 2024-09-15 20:11:08 浏览: 52
在C语言中,文件I/O(Input/Output)操作用于从磁盘或其他存储设备读取或写入数据。为了保存账户信息,你需要使用标准库中的`stdio.h`头文件,特别是其中的`fopen()`, `fprintf()`, 和 `fclose()` 函数。
以下是一个简单的示例,展示了如何创建一个文本文件并保存账户信息(例如用户名和密码):
```c
#include <stdio.h>
// 假设我们有一个结构体表示账户信息
typedef struct {
char username[50];
char password[50];
} Account;
void save_account_info(Account account, const char* filename) {
FILE *file; // 文件指针
// 打开文件(如果不存在则创建)
if ((file = fopen(filename, "w")) == NULL) {
perror("Error opening file");
return;
}
// 写入账户信息
fprintf(file, "%s\n", account.username);
fprintf(file, "%s\n", account.password);
// 关闭文件
fclose(file);
}
int main() {
Account user = {"Alice", "123456"};
save_account_info(user, "accounts.txt");
return 0;
}
```
在这个例子中:
- `fopen()`函数尝试打开指定的文件名,第一个参数是文件名,第二个参数是模式字符串,"w"表示写入模式,如果文件不存在则创建。
- `fprintf(file, ...)`用于格式化输出到文件,`\n`用于换行。
- `fclose(file)`确保文件在不再需要时被正确关闭。
阅读全文
相关推荐
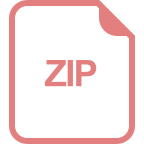
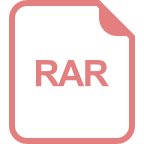
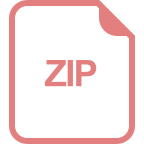
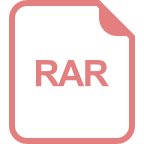
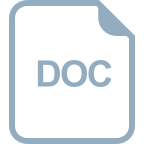
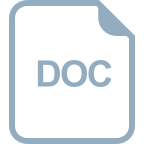
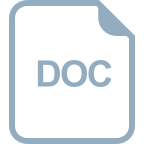
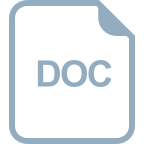
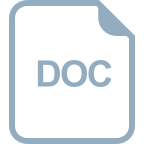
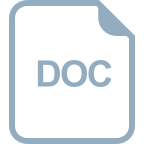
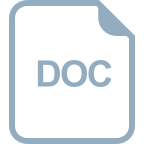
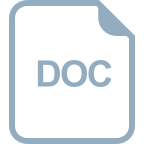
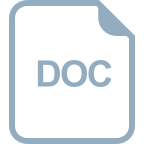
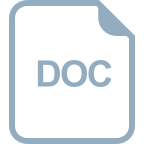
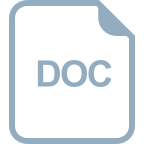
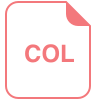


