用python程序寻找目标像素
时间: 2024-10-06 15:05:20 浏览: 29
在Python中,要找到图像中的特定像素,通常可以使用PIL(Python Imaging Library)或者其更现代的替代品Pillow库。以下是一个基本步骤:
1. 首先,你需要导入`Pillow`库,并打开包含目标像素的图片文件。
```python
from PIL import Image
image = Image.open('your_image.jpg')
```
2. 然后,你可以通过索引来访问像素。每个像素在图像中由一个坐标表示,例如(行, 列)。假设你想找到红色像素(通常用RGB值来表示,如(255, 0, 0)),你可以遍历图像矩阵来查找匹配的颜色。
```python
target_color = (255, 0, 0)
found_pixels = []
for pixel in image.getdata():
if pixel == target_color:
found_pixels.append(pixel)
```
3. 如果找到了目标像素,`found_pixels`列表将包含所有匹配的位置。
如果你需要精确地定位单个像素,还可以使用`crop`或`getpixel`函数。例如:
```python
x, y = find_pixel_coordinate(image, target_color) # 这里是根据算法或条件找到坐标的方法
target_pixel = image.getpixel((x, y))
```
请注意,这个过程可能会消耗较多计算资源,特别是对于大图像。
相关问题
用python程序高效实现寻找目标像素群
在Python中,要高效地寻找图像中的目标像素群,通常会使用图像处理库如PIL(Python Imaging Library)或OpenCV。这里是一个简单步骤的例子:
1. 导入所需库:
```python
from PIL import Image
import numpy as np
```
2. 加载图片并转换为numpy数组:
```python
img = Image.open('your_image.jpg')
image_array = np.array(img)
```
3. 定义目标像素的颜色范围或阈值(例如,如果目标是红色区域):
```python
target_color_min = (0, 0, 255) # 红色的最低RGB值
target_color_max = (5, 5, 255) # 红色的最高RGB值
```
4. 使用`inrange()`函数筛选出目标颜色范围内的像素:
```python
mask = np.all(image_array >= target_color_min, axis=-1) & np.all(image_array <= target_color_max, axis=-1)
```
这将创建一个二进制掩码,其中白色像素表示目标颜色。
5. 获取目标像素位置:
```python
target_pixels = np.argwhere(mask)
```
`target_pixels`现在是一个二维数组,包含了所有匹配的目标像素坐标。
6. 可视化结果:
```python
new_img = image_array.copy()
new_img[~mask] = [0, 0, 0] # 将非目标区域设为黑色
display(new_img)
```
用python程序高效实现寻找纹理目标像素群
在Python中,使用图像处理库如OpenCV和PIL来寻找纹理目标像素群通常涉及以下几个步骤:
1. **导入所需库**:首先需要导入`cv2`(OpenCV的Python接口)以及可能用于数据操作的`numpy`。
```python
import cv2
import numpy as np
```
2. **读取图片**:使用`cv2.imread()`函数加载图像,并转换为灰度图像以便更好地分析纹理特征。
```python
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
```
3. **预处理**:对图像进行平滑处理,例如高斯滤波,可以使用`cv2.GaussianBlur()`。
```python
img_blur = cv2.GaussianBlur(img, (5, 5), 0)
```
4. **计算纹理特征**:常用的纹理特征有灰度共生矩阵(GLCM)、局部二值模式(LBP)等。对于GLCM,可以使用`skimage.feature.greycomatrix()`;对于LBP,`skimage.feature.local_binary_pattern()`。
```python
from skimage.feature import greycomatrix, local_binary_pattern
# 使用GLCM
lcm = greycomatrix(img_blur, distances=[1], angles=[0], levels=256)
# 或者使用LBP
lbp = local_binary_pattern(img, P=8, R=1, method='uniform')
```
5. **阈值处理**:通过阈值分割将纹理部分从背景中分离出来。可以使用`cv2.threshold()`函数。
```python
_, texture_mask = cv2.threshold(lbp, threshold, 255, cv2.THRESH_BINARY)
```
6. **查找目标像素群**:最后,你可以使用`np.nonzero()`找出非零像素(即目标纹理区域),得到像素坐标数组。
```python
target_pixels = np.argwhere(texture_mask)
```
阅读全文
相关推荐
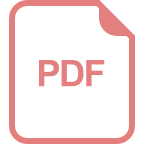
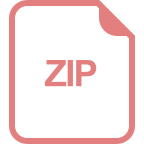
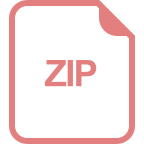
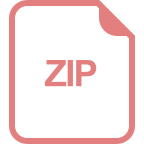
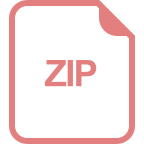
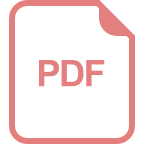
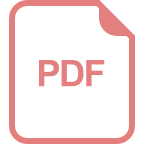
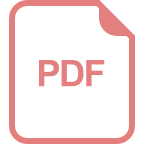
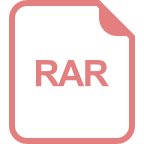
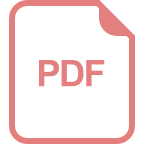
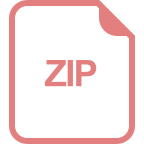
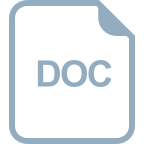
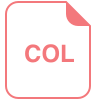
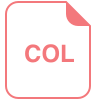
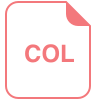

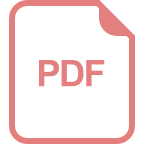
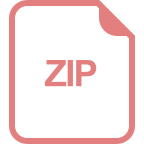