如何使用subprocess模块在PyQt5中处理非阻塞式执行?
时间: 2024-10-25 20:17:33 浏览: 15
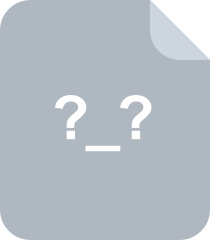
PyQt5中异步刷新UI+Python中的多线程+python中使用subprocess总结
在PyQt5中,当你需要使用`subprocess`模块执行命令而不阻塞主线程时,可以借助`QThread`或者`Popen.communicate()`配合回调函数来实现异步非阻塞执行。以下是步骤:
1. **使用QThread**:
- 首先,创建一个`QThread`对象,它是处理子进程执行的地方。
- 在`__init__`中,创建`subprocess.Popen`实例,并设置`shell=True`(如果需要执行的是 shell 命令)。
- 将`Popen`的`stdout`和`stderr`连接到`QThread`的`signals`槽函数中,比如`readyReadStandardOutput`和`readyReadStandardError`。
- 在`QThread`中定义槽函数,如`read_output`,负责读取子进程的输出并处理结果。
- 启动线程,例如`thread.start()`
- 在主线程中,使用`thread.quit()`或`thread.wait()`等待子线程完成后再继续。
```python
from PyQt5.QtCore import pyqtSignal, QThread
import subprocess
class WorkerThread(QThread):
outputSignal = pyqtSignal(str)
def __init__(self, command):
super().__init__()
self.command = command
def run(self):
process = subprocess.Popen(self.command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
while True:
output = process.stdout.readline().decode()
error = process.stderr.readline().decode()
if not output and not error:
break
# 发送信号到主线程
self.outputSignal.emit(output)
self.outputSignal.emit(error)
# 等待子进程完成
process.wait()
# 在主窗口中使用
command = "your_command_here"
worker = WorkerThread(command)
worker.outputSignal.connect(self.handle_output) # 这里假设handle_output是一个槽函数处理输出
worker.finished.connect(worker.deleteLater) # 当线程完成后删除
worker.start()
```
2. **使用`communicate()`配合回调**:
- 直接在主线程中创建`Popen`,但是每次调用`communicate()`时,将其结果放入一个队列或信号槽。
- 在`communicate()`结束后,检查结果并进行相应操作。
```python
def execute_nonblocking(command):
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE)
output_queue = queue.Queue()
error_queue = queue.Queue()
def read_and_enqueue(stream, queue):
for line in iter(stream.readline, b''):
decoded_line = line.decode()
if decoded_line:
queue.put(decoded_line)
thread_stdout = threading.Thread(target=read_and_enqueue, args=(process.stdout, output_queue))
thread_stderr = threading.Thread(target=read_and_enqueue, args=(process.stderr, error_queue))
thread_stdout.start()
thread_stderr.start()
process.wait()
thread_stdout.join()
thread_stderr.join()
return (output_queue.get(), error_queue.get())
# 使用回调函数处理结果
output, error = execute_nonblocking("your_command_here")
if error:
handle_error(error)
else:
handle_output(output)
```
阅读全文
相关推荐
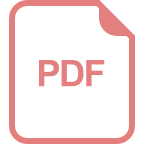
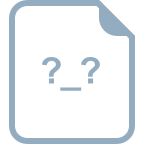

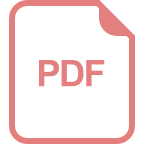














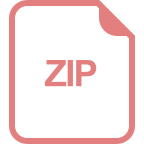