如何编写一个自定义函数 myrange,它能够模拟 Python 的内置 range 函数的功能?
时间: 2024-12-15 19:19:54 浏览: 5
要编写一个自定义函数 `myrange` 来模拟 Python 内置的 `range` 函数,你可以创建一个函数,接受三个参数 start, stop, step,然后通过一个循环返回一系列指定范围内的整数序列。以下是简单的 Python 实现:
```python
def myrange(start, stop=None, step=1):
if stop is None:
stop = start + 1 # 如果只提供了一个参数,假设它是指定的终点
if step > 0:
for i in range(start, stop, step):
yield i
elif step < 0:
for i in range(start, stop - 1, -step): # 步长为负时调整循环方向
yield i
else:
raise ValueError("Step must not be zero for a valid range.")
# 示例用法
for num in myrange(0, 10, 2): # 返回 0, 2, 4, 6, 8
print(num)
相关问题
本关任务:实现一个与 Python 内置函数range相似的函数myrange
在Python中,内置函数`range()`可以生成一个整数序列。自定义一个与`range()`相似的函数`myrange()`可以通过实现一个生成器来完成。下面是一个简单的`myrange`函数实现的例子:
```python
def myrange(start, stop, step=1):
if step == 0:
raise ValueError("step 参数不能为0")
if step > 0 and start >= stop:
return
elif step < 0 and start <= stop:
return
if step > 0:
while start < stop:
yield start
start += step
else:
while start > stop:
yield start
start += step
```
这个函数接受三个参数:`start`(起始值),`stop`(结束值,但不包括在生成的序列中),以及`step`(步长,默认为1)。函数通过一个`while`循环和`yield`语句生成一个序列,并且在每次迭代中返回序列中的下一个值。需要注意的是,如果`step`为0,函数将抛出`ValueError`异常,因为步长为零没有任何意义。
Python实现: 利用迭代器的原理构造一个具有与range()相同功能的迭代器。命名为myrange类 利用迭代器的原理构造一个具有与zip()相同功能的迭代器,命名为myzip类
好的,我会用 Python 实现。
首先是 `myrange` 类:
```python
class myrange:
def __init__(self, start, stop=None, step=1):
if stop is None:
stop = start
start = 0
self.start = start
self.stop = stop
self.step = step
self.current = start
def __iter__(self):
return self
def __next__(self):
if self.step > 0 and self.current >= self.stop:
raise StopIteration
elif self.step < 0 and self.current <= self.stop:
raise StopIteration
else:
result = self.current
self.current += self.step
return result
```
`myrange` 类接受与 `range()` 函数相同的参数,即起始值、终止值和步长。它的 `__iter__` 方法返回自身,因此它是可迭代的。`__next__` 方法计算下一个值并返回它,直到达到终止值或步长为零。
接下来是 `myzip` 类:
```python
class myzip:
def __init__(self, *args):
self.iterators = [iter(arg) for arg in args]
def __iter__(self):
return self
def __next__(self):
try:
return tuple(next(iterator) for iterator in self.iterators)
except StopIteration:
raise StopIteration
```
`myzip` 类接受任意数量的可迭代对象,并为每个对象创建一个迭代器。它的 `__iter__` 方法返回自身,因此它是可迭代的。`__next__` 方法从每个迭代器中取出一个元素,并返回一个元组,直到任何一个迭代器抛出 `StopIteration` 异常。此时,它也抛出 `StopIteration` 异常以终止迭代。
使用这两个类的例子:
```python
# 使用 myrange
for i in myrange(5):
print(i) # 0 1 2 3 4
# 使用 myzip
a = [1, 2, 3]
b = ['a', 'b', 'c']
for x, y in myzip(a, b):
print(x, y) # 1 a 2 b 3 c
```
阅读全文
相关推荐
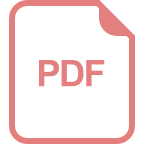
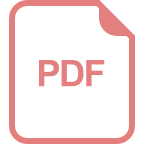
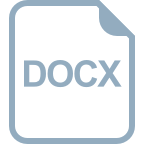
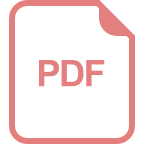
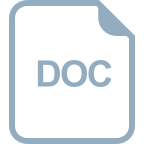
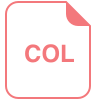
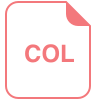
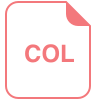
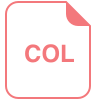
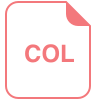
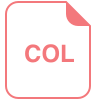
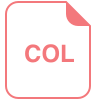
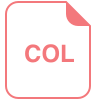



