labelme下载代码
时间: 2024-05-25 11:08:42 浏览: 153
LabelMe是一个在线的半自动标注工具,可以用于对图像进行标注,同时也可以用于标注视频。如果您需要将这个工具应用到自己的项目中,您可以访问官方网站下载代码并进行修改。以下是LabelMe的下载方法:
1. 首先,您需要访问LabelMe的官方网站:http://labelme.csail.mit.edu/Release3.0/
2. 在页面底部找到“Download”按钮,点击进入下载页面。
3. 在下载页面中,您可以选择下载源代码或者已经编译好的版本。如果您需要进行修改或者添加新的功能,建议下载源代码。
4. 根据您的需求选择合适的版本进行下载,下载完成后解压缩即可使用。
5. 如果您需要使用LabelMe进行图像标注,请确保您已经安装了Python和相关的依赖库。如果您需要使用LabelMe进行视频标注,则还需要安装FFmpeg等相关工具。
相关问题
labelme裁剪代码
你可以使用 Labelme 工具来进行图像的裁剪。Labelme是一个用于图像标注的开源工具,它可以帮助你创建和编辑图像标注数据。
下面是一个示例代码,演示如何使用 Labelme 进行图像的裁剪:
```python
import labelme
import json
from PIL import Image
def crop_image(image_path, annotation_path, output_path):
# 打开图像
image = Image.open(image_path)
# 加载标注数据
with open(annotation_path, 'r') as f:
annotation = json.load(f)
# 获取裁剪区域
shapes = annotation['shapes']
for shape in shapes:
if shape['shape_type'] == 'rectangle':
x, y, w, h = shape['points'][0] + shape['points'][1]
cropped_image = image.crop((x, y, x + w, y + h))
cropped_image.save(output_path)
# 示例用法
image_path = 'input.jpg'
annotation_path = 'input.json'
output_path = 'output.jpg'
crop_image(image_path, annotation_path, output_path)
```
在上面的示例中,需要提供原始图像的路径(`image_path`),以及通过 Labelme 标注得到的标注文件的路径(`annotation_path`)。代码将根据标注文件中的矩形框坐标信息对图像进行裁剪,并将裁剪结果保存到指定的输出路径(`output_path`)。
请确保在使用代码之前,已经安装了必要的依赖库,如 labelme、Pillow 等。你可以根据自己的需求进行修改和扩展。
用qt实现labelme的代码
Labelme是一个用于图像标注的开源工具,使用Python和Qt开发。下面是一个简单的实现Labelme的代码示例:
```python
import os
import json
from PyQt5.QtCore import Qt, QPoint, QRect
from PyQt5.QtGui import QImage, QPixmap, QPainter, QPen
from PyQt5.QtWidgets import QApplication, QMainWindow, QGraphicsScene, QGraphicsView, QGraphicsPixmapItem, QAction, QFileDialog
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.image_path = ''
self.annotations = []
self.current_annotation = None
self.current_polygon = []
self.scene = QGraphicsScene(self)
self.view = QGraphicsView(self.scene)
self.view.setDragMode(QGraphicsView.ScrollHandDrag)
self.view.setRenderHint(QPainter.Antialiasing)
self.setCentralWidget(self.view)
self.create_actions()
self.create_menus()
self.setWindowTitle('Labelme')
def create_actions(self):
self.open_image_action = QAction('Open Image', self)
self.open_image_action.setShortcut('Ctrl+O')
self.open_image_action.triggered.connect(self.open_image)
self.save_annotations_action = QAction('Save Annotations', self)
self.save_annotations_action.setShortcut('Ctrl+S')
self.save_annotations_action.triggered.connect(self.save_annotations)
self.quit_action = QAction('Quit', self)
self.quit_action.setShortcut('Ctrl+Q')
self.quit_action.triggered.connect(self.close)
def create_menus(self):
self.file_menu = self.menuBar().addMenu('File')
self.file_menu.addAction(self.open_image_action)
self.file_menu.addAction(self.save_annotations_action)
self.file_menu.addAction(self.quit_action)
def open_image(self):
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_path, _ = QFileDialog.getOpenFileName(self, 'Open Image', '', 'Images (*.png *.xpm *.jpg *.bmp *.gif)', options=options)
if file_path:
self.image_path = file_path
self.scene.clear()
self.current_annotation = None
self.current_polygon = []
pixmap = QPixmap(file_path)
item = QGraphicsPixmapItem(pixmap)
self.scene.addItem(item)
self.view.fitInView(item, Qt.KeepAspectRatio)
def save_annotations(self):
if not self.image_path:
return
annotations_file = os.path.splitext(self.image_path)[0] + '.json'
with open(annotations_file, 'w') as f:
json.dump(self.annotations, f)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
if not self.current_annotation:
self.current_annotation = {
'image_path': self.image_path,
'annotations': []
}
self.annotations.append(self.current_annotation)
self.current_polygon.append(self.view.mapToScene(event.pos()))
if len(self.current_polygon) > 1:
self.scene.addLine(QLineF(self.current_polygon[-2], self.current_polygon[-1]), QPen(Qt.red))
def keyPressEvent(self, event):
if event.key() == Qt.Key_Return:
if self.current_polygon:
self.current_annotation['annotations'].append(self.current_polygon)
self.current_polygon = []
self.scene.clear()
pixmap = QPixmap(self.image_path)
item = QGraphicsPixmapItem(pixmap)
self.scene.addItem(item)
self.view.fitInView(item, Qt.KeepAspectRatio)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
这个示例代码实现了打开图片、绘制多边形标注、保存标注等功能。但是这只是一个简单的示例,实际使用中还需要考虑更多的细节和功能。
阅读全文
相关推荐
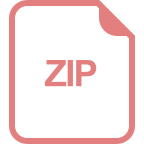
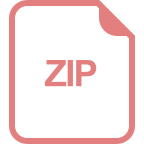





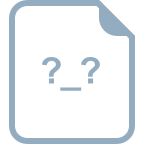
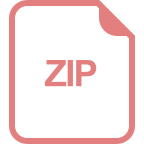
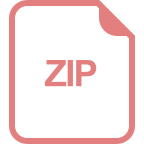
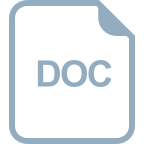



