实现简单通讯录的Android Studio 完整代码
时间: 2023-11-27 19:48:08 浏览: 68
以下是一个简单通讯录的Android Studio完整代码,包括布局文件和Java代码:
1.布局文件activity_main.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/editTextName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Name" />
<EditText
android:id="@+id/editTextPhone"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Phone" />
<Button
android:id="@+id/buttonAdd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Add" />
<ListView
android:id="@+id/listViewContacts"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
```
2.布局文件list_item_contact.xml:
```xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/textViewName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Name" />
<TextView
android:id="@+id/textViewPhone"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Phone" />
</LinearLayout>
```
3.Java代码MainActivity.java:
```java
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
private EditText editTextName, editTextPhone;
private Button buttonAdd;
private ListView listViewContacts;
private ArrayList<Contact> contactList;
private ContactListAdapter contactListAdapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
editTextName = findViewById(R.id.editTextName);
editTextPhone = findViewById(R.id.editTextPhone);
buttonAdd = findViewById(R.id.buttonAdd);
listViewContacts = findViewById(R.id.listViewContacts);
contactList = new ArrayList<>();
contactListAdapter = new ContactListAdapter(this, R.layout.list_item_contact, contactList);
listViewContacts.setAdapter(contactListAdapter);
buttonAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String name = editTextName.getText().toString();
String phone = editTextPhone.getText().toString();
if (name.isEmpty() || phone.isEmpty()) {
Toast.makeText(MainActivity.this, "Please enter name and phone", Toast.LENGTH_SHORT).show();
} else {
Contact contact = new Contact(name, phone);
contactList.add(contact);
contactListAdapter.notifyDataSetChanged();
editTextName.setText("");
editTextPhone.setText("");
}
}
});
}
}
```
相关推荐
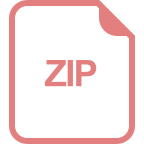
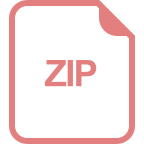
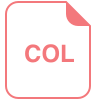













