sh脚本 获取函数返回值
时间: 2024-06-09 16:05:49 浏览: 25
在Shell脚本中,可以使用以下方法获取函数的返回值:
1. 使用$?变量:在函数调用后,$?变量会保存函数的返回值。例如:
```bash
#!/bin/bash
# 定义一个函数
my_function() {
echo "Hello, World!"
return 42
}
# 调用函数
my_function
# 获取返回值
result=$?
echo "返回值为: $result"
```
输出结果会是:返回值为: 42。
2. 使用命令替换:通过将函数调用放在$(...)内,可以直接获取函数的返回值。例如:
```bash
#!/bin/bash
# 定义一个函数
my_function() {
echo "Hello, World!"
return 42
}
# 获取返回值
result=$(my_function)
echo "返回值为: $result"
```
输出结果同样是:返回值为: 42。
这些方法都可以用来获取函数的返回值,你可以选择适合你需求的方式来使用。
相关问题
java获取shell脚本返回值
Java可以通过Process类执行Shell脚本,并通过该类的waitFor()方法获取Shell脚本执行的返回值。
示例代码如下:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class ShellExecute {
public static void main(String[] args) throws IOException, InterruptedException {
String command = "sh /path/to/script.sh";
Process process = Runtime.getRuntime().exec(command);
int exitCode = process.waitFor();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
if (exitCode == 0) {
System.out.println("Shell脚本执行成功!");
} else {
System.out.println("Shell脚本执行失败,返回值:" + exitCode);
}
}
}
```
其中,command变量存储要执行的Shell脚本的命令,process.waitFor()方法会等待脚本执行完成并返回脚本的退出状态码。如果退出状态码为0,则代表脚本执行成功。如果不为0,则代表脚本执行失败,并可以根据具体的状态码进行处理。通过BufferedReader类读取脚本的输出信息。
获取linux shell脚本返回值
在Linux shell脚本中,可以通过$?来获取脚本的返回值。具体来说,$?保存了上一个命令或函数的退出状态码。在函数中,可以使用return语句来指定函数的返回值,该返回值会保存在$?中。但需要注意的是,$?会在函数返回后很快变回0值,所以应在函数返回后尽快将$?保存在变量中,然后通过该变量来访问返回值,而不是直接通过$?来进行后续处理。\[1\]
在Linux中,规定正常退出的命令和脚本都应该以0作为其返回值,而任何非0的返回值都表示命令未正确退出或未正常执行。因此,可以通过判断$?的值是否为0来判断命令或脚本是否执行成功。\[2\]
举个例子,假设有一个脚本test.sh,执行后会输出一些信息并执行一个命令ehco(错误的命令)。在脚本执行完毕后,可以通过$?来获取脚本的返回值。在这个例子中,脚本的返回值为127,表示命令未找到。\[3\]
#### 引用[.reference_title]
- *1* [linux shell函数返回值问题](https://blog.csdn.net/y24283648/article/details/124655540)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [linux获取脚本或命令返回值](https://blog.csdn.net/dingding_ting/article/details/116458178)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insertT0,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
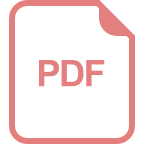
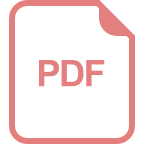












