用cpp,包括有整数的比较、浮点数的比较、双精度的比较。 先有一个判断比较的类型,如果输入1,则为整数;2为浮点数;3为双精度。
时间: 2024-10-21 11:09:20 浏览: 25
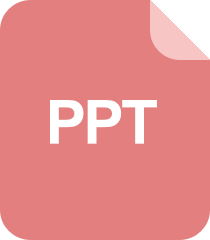
浮点数的精度和有效位-钱能C++程序设计教程(第二版)课件\\C++程序设计教程4
在C++中,你可以创建一个函数来处理不同类型的数据比较,通过条件语句判断输入的类型并选择相应的比较操作。这里是一个简单的示例:
```cpp
#include <iostream>
#include <typeinfo>
// 比较函数模板,用于整数、浮点数和双精度数
template <typename T>
bool compare(T a, T b) {
// 使用typeid来检查数据类型
if constexpr (std::is_integral<T>::value) {
// 整数比较
return a == b;
} else if constexpr (std::is_floating_point<T>::value) {
// 浮点数和双精度数比较
static_assert(std::numeric_limits<T>::is_iec559, "Floating point type not supported");
return std::abs(a - b) < std::numeric_limits<T>::epsilon();
} else {
throw std::runtime_error("Unsupported data type for comparison");
}
}
int main() {
int int_val = 0;
float float_val = 0.0f;
double double_val = 0.0;
// 获取用户输入
int comparison_type;
std::cout << "Enter the comparison type (1: integer, 2: floating point, 3: double): ";
std::cin >> comparison_type;
switch (comparison_type) {
case 1: // 整数比较
std::cout << "Comparing integers: " << (compare(int_val, int_val) ? "equal" : "not equal") << std::endl;
break;
case 2: // 浮点数比较
std::cout << "Comparing floats: " << (compare(float_val, float_val) ? "equal" : "not equal") << std::endl;
break;
case 3: // 双精度数比较
std::cout << "Comparing doubles: " << (compare(double_val, double_val) ? "equal" : "not equal") << std::endl;
break;
default:
std::cerr << "Invalid input! Comparison not performed." << std::endl;
break;
}
return 0;
}
```
这个程序会根据用户的输入来选择比较类型,并使用`compare`函数来进行实际的比较。注意,对于浮点数的比较,我们使用了`std::numeric_limits<T>::epsilon()`来检测两个值是否足够接近,通常认为它们相等。
阅读全文
相关推荐
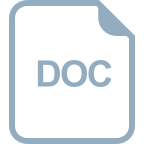
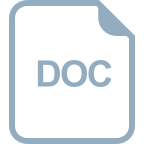













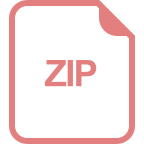

