汉诺塔的迭代与递归算法实现
时间: 2024-09-18 20:11:47 浏览: 35
汉诺塔是一个经典的计算机科学问题,涉及三个柱子和一些大小不同的圆盘。目标是从第一个柱子将所有盘子移动到第三个柱子,过程中任何时候都只能将比当前大盘子小的盘子放在大盘子上面。这里提供一种简单的递归和迭代的实现:
**递归算法**:
```python
def hanoi(n, source, auxiliary, target):
if n > 0:
# 递归地处理前n - 1, source, target, auxiliary)
# 将最大的盘子从源移到目标
print(f"Move disk {n} from {source} to {target}")
# 最后把辅助柱子上剩余的盘子移到目标
hanoi(n - 1, auxiliary, source, target)
# 调用函数,例如有3个盘子
hanoi(3, 'A', 'B', 'C')
```
**迭代算法** (也称为循环法):
```python
def hanoi_iterative(n, source, auxiliary, target):
stacks = [[source, []], [auxiliary, []], [target, []]] # 初始化三堆栈
def move_disk(from_stack, to_stack):
top_disk, aux = stacks[from_stack][1], stacks[to_stack][1]
if aux: # 如果to_stack非空,先移动其顶部盘子
move_disk(to_stack, aux)
stacks[from_stack][1].pop() # 移动源堆栈的顶盘
stacks[to_stack][0].append(top_disk) # 放入目标堆栈
if aux: # 再次检查是否需要移动from_stack的剩余盘子
move_disk(from_stack, aux)
# 主循环
for _ in range(2 ** n - 1):
move_disk(stacks[0], stacks[2]) # 双层循环,完成一次移动
# 同样调用函数
hanoi_iterative(3, 'A', 'B', 'C')
```
阅读全文
相关推荐
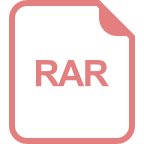
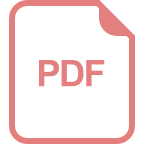
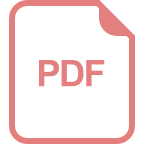









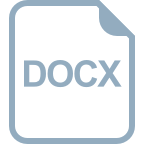
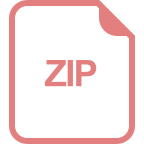
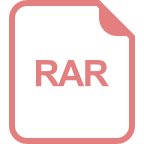
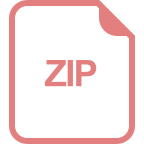
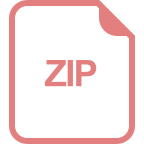
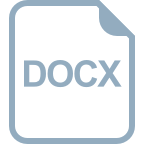