2、编写一个多文件C程序项目,要求从键盘输入两个整数,主函数从项目的其它文件中调用四则运算函数,并能够将计算结果输出。
时间: 2024-12-26 17:31:09 浏览: 1
在C语言中,创建一个多文件项目通常涉及以下几个步骤:
1. **创建目录结构**:首先,你可以创建一个包含三个文件的简单目录结构:`main.c` (主文件), `calculator.c` (包含四则运算函数) 和 `calculator.h` (声明头文件)。
```markdown
my_project/
├── main.c
├── calculator.c
└── calculator.h
```
2. **在`calculator.h`中声明函数**:在`calculator.h`里,你需要声明四则运算函数,例如 `add`, `subtract`, `multiply`, 和 `divide`。例如:
```c
// calculator.h
#ifndef CALCULATOR_H
#define CALCULATOR_H
#include <stdio.h>
int add(int a, int b);
int subtract(int a, int b);
int multiply(int a, int b);
int divide(int a, int b);
#endif // CALCULATOR_H
```
3. **在`calculator.c`中实现函数**:在`calculator.c` 文件里,定义并实现这些函数:
```c
// calculator.c
#include "calculator.h"
int add(int a, int b) {
return a + b;
}
int subtract(int a, int b) {
return a - b;
}
int multiply(int a, int b) {
return a * b;
}
int divide(int a, int b) {
if (b != 0) {
return a / b;
} else {
printf("Error: Division by zero is not allowed.\n");
return 0; // 或者抛出异常
}
}
```
4. **在`main.c`中导入并使用函数**:在`main.c` 中,首先包含`calculator.h`,然后从`calculator.c`中链接并调用这些函数,获取用户输入并显示结果:
```c
// main.c
#include "calculator.h"
#include <stdio.h>
#include <stdlib.h>
int main() {
int num1, num2;
printf("Enter two integers: ");
scanf("%d %d", &num1, &num2);
int result = add(num1, num2); // 使用示例
printf("Addition: %d\n", result);
result = subtract(num1, num2);
printf("Subtraction: %d\n", result);
result = multiply(num1, num2);
printf("Multiplication: %d\n", result);
if (result != 0) {
result = divide(num1, num2);
printf("Division: %.1f\n", (double)result);
}
return 0;
}
```
5. **编译和运行**:在命令行中,使用`gcc`或其他C编译器(如`clang`),指定源文件的位置以及链接库文件(-l)来编译项目:
```sh
gcc -o main main.c calculator.c -I. # -I. 表示当前目录下有头文件
./main
```
运行这个程序,它会从键盘接收输入,进行四则运算,并打印结果。
阅读全文
相关推荐
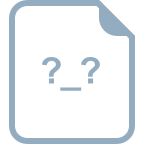
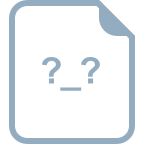
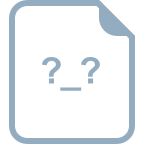



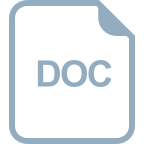
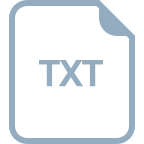
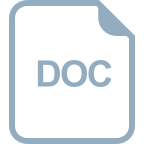
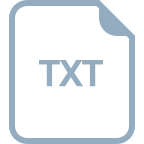
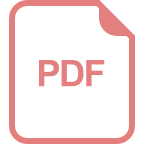
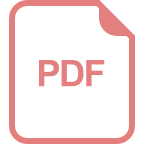
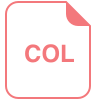
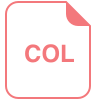
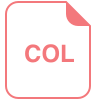
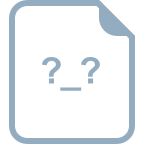
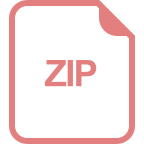
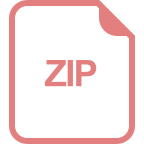
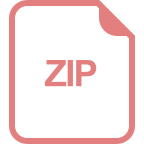