springboot nio读取文件
时间: 2023-08-15 17:14:14 浏览: 75
在 Spring Boot 中使用 NIO(New Input/Output)读取文件可以通过以下步骤实现:
1. 导入相关的依赖:在 Maven 或 Gradle 中添加对 `spring-boot-starter-web` 的依赖。
2. 创建一个 `RestController` 类,用于处理 HTTP 请求。
3. 在该类中创建一个处理文件读取请求的接口,可以使用 `@GetMapping` 注解来指定接口的路径和请求方法。
4. 在接口方法中使用 `Path` 和 `Files` 类来读取文件。可以使用 `Paths.get()` 方法获取文件路径,并使用 `Files.readAllBytes()` 方法读取文件内容。
5. 将文件内容以字符串形式返回给客户端。
下面是一个简单的示例:
```java
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
@RestController
public class FileController {
@GetMapping("/read-file")
public String readFile() {
String filePath = "path/to/your/file.txt";
try {
Path path = Paths.get(filePath);
byte[] fileContent = Files.readAllBytes(path);
return new String(fileContent);
} catch (IOException e) {
e.printStackTrace();
return "Error reading file";
}
}
}
```
请注意,上述示例中的文件路径需要替换为你实际的文件路径。你可以通过访问 `/read-file` 路径来读取文件内容。
相关推荐
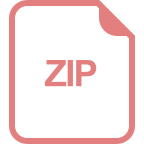
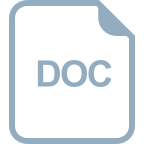














