Python15个超炫酷的代码
时间: 2024-09-29 17:01:36 浏览: 17
Python作为一种简洁而强大的编程语言,有许多实用且优雅的代码示例。这里列举一些常见的、超炫酷的Python代码片段:
1. 列表推导式(List Comprehension)生成新列表:
```python
squares = [x**2 for x in range(10)]
```
2. 生成器表达式(Generator Expression)节省内存:
```python
even_squares = (x**2 for x in range(10) if x % 2 == 0)
```
3. 装饰器(Decorator)修改函数行为:
```python
def my_decorator(func):
def wrapper():
print("Before function call")
func()
print("After function call")
return wrapper
@my_decorator
def say_hello():
print("Hello")
say_hello()
```
4. 使用`zip()`和`*`操作符同时遍历多个序列:
```python
names, ages = ['Alice', 'Bob', 'Charlie'], [20, 22, 25]
for name, age in zip(names, ages):
print(f"{name} is {age} years old.")
```
5. 空间内建常量和函数(如空字符串`''`,None值等):
```python
empty_list = []
None_value = None
```
6. 使用Python的标准库函数高阶编程(例如`map()`, `filter()`):
```python
numbers = list(map(lambda x: x * x, range(1, 6)))
```
相关推荐
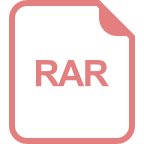
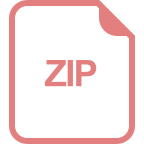
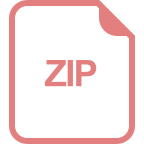














