python实现bmp图像处理
时间: 2023-10-23 12:11:34 浏览: 329
要使用Python实现对BMP图像的处理,可以按照以下步骤进行:
1. 读取BMP图像:将BMP图像文件作为二进制文件读取并解析其文件头和像素数据。
2. 调整图像大小(Resize):使用插值算法(例如最近邻插值、双线性插值)计算新图像中每个像素的值,并生成调整后的图像。
3. 旋转图像(Rotate):通过对图像进行几何变换,将像素从原始位置移动到新的位置来实现旋转效果。
4. 保存BMP图像:将处理后的图像数据写入新的BMP文件中,并确保文件头和像素数据的正确格式。
下面是完整的Python代码示例,展示了如何实现对BMP图像的处理:
```python
import struct
# 读取BMP图像
def read_bmp_image(file_path):
with open(file_path, 'rb') as f:
# 解析文件头
file_header = f.read(14)
# 解析图像信息头
info_header = f.read(40)
# 获取图像的宽度和高度
width = struct.unpack('<i', info_header[4:8])[0]
height = struct.unpack('<i', info_header[8:12])[0]
# 读取像素数据
pixel_data = f.read()
return file_header + info_header + pixel_data, width, height
# 调整图像大小
def resize_image(image_data, width, height, new_width, new_height):
# 实现图像大小的调整算法
return resized_image_data, new_width, new_height
# 旋转图像
def rotate_image(image_data, width, height, angle):
# 实现图像旋转算法
return rotated_image_data, new_width, new_height
# 保存BMP图像
def save_bmp_image(file_path, image_data, width, height):
with open(file_path, 'wb') as f:
f.write(image_data)
print('BMP图像已保存至', file_path)
# 读取BMP图像
image_data, width, height = read_bmp_image('input.bmp')
# 调整图像大小
resized_image_data, new_width, new_height = resize_image(image_data, width, height, 640, 480)
# 旋转图像
rotated_image_data, new_width, new_height = rotate_image(resized_image_data, new_width, new_height, 90)
# 保存BMP图像
save_bmp_image('output.bmp', rotated_image_data, new_width, new_height)
```
一些
阅读全文
相关推荐
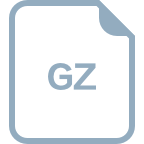
















