jsp+简易图书管理系统
时间: 2023-12-10 18:34:55 浏览: 147
以下是一个简单的基于JSP和Servlet的图书管理系统的实现,包括添加、删除、修改和查询图书信息的功能:
1. 首先创建一个名为“book”的JavaBean类,用于存储图书信息,包括图书编号、图书名称、作者和价格等属性。
```java
public class Book {
private String id;
private String name;
private String author;
private double price;
// 构造方法
public Book(String id, String name, String author, double price) {
this.id = id;
this.name = name;
this.author = author;
this.price = price;
}
// getter和setter方法
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
```
2. 创建一个名为“BookDao”的Java类,用于实现对图书信息的增删改查操作,包括添加图书、删除图书、修改图书和查询图书等方法。
```java
public class BookDao {
// 存储图书信息的List
private static List<Book> bookList = new ArrayList<>();
// 添加图书
public static void addBook(Book book) {
bookList.add(book);
}
// 删除图书
public static void deleteBook(String id) {
for (Book book : bookList) {
if (book.getId().equals(id)) {
bookList.remove(book);
break;
}
}
}
// 修改图书
public static void updateBook(Book book) {
for (Book b : bookList) {
if (b.getId().equals(book.getId())) {
b.setName(book.getName());
b.setAuthor(book.getAuthor());
b.setPrice(book.getPrice());
break;
}
}
}
// 查询图书
public static Book getBook(String id) {
for (Book book : bookList) {
if (book.getId().equals(id)) {
return book;
}
}
return null;
}
// 获取所有图书
public static List<Book> getAllBooks() {
return bookList;
}
}
```
3. 创建一个名为“BookServlet”的Servlet类,用于处理客户端的请求,包括添加图书、删除图书、修改图书和查询图书等操作。
```java
@WebServlet("/book")
public class BookServlet extends HttpServlet {
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String action = request.getParameter("action");
if ("add".equals(action)) {
addBook(request, response);
} else if ("delete".equals(action)) {
deleteBook(request, response);
} else if ("update".equals(action)) {
updateBook(request, response);
} else if ("get".equals(action)) {
getBook(request, response);
} else if ("getAll".equals(action)) {
getAllBooks(request, response);
}
}
// 添加图书
private void addBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String id = request.getParameter("id");
String name = request.getParameter("name");
String author = request.getParameter("author");
double price = Double.parseDouble(request.getParameter("price"));
Book book = new Book(id, name, author, price);
BookDao.addBook(book);
response.sendRedirect("book.jsp");
}
// 删除图书
private void deleteBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String id = request.getParameter("id");
BookDao.deleteBook(id);
response.sendRedirect("book.jsp");
}
// 修改图书
private void updateBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String id = request.getParameter("id");
String name = request.getParameter("name");
String author = request.getParameter("author");
double price = Double.parseDouble(request.getParameter("price"));
Book book = new Book(id, name, author, price);
BookDao.updateBook(book);
response.sendRedirect("book.jsp");
}
// 查询图书
private void getBook(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String id = request.getParameter("id");
Book book = BookDao.getBook(id);
request.setAttribute("book", book);
request.getRequestDispatcher("book.jsp").forward(request, response);
}
// 获取所有图书
private void getAllBooks(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
List<Book> bookList = BookDao.getAllBooks();
request.setAttribute("bookList", bookList);
request.getRequestDispatcher("book.jsp").forward(request, response);
}
}
```
4. 创建一个名为“book.jsp”的JSP页面,用于显示图书信息和处理客户端的请求。
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>图书管理系统</title>
</head>
<body>
<h1>图书管理系统</h1>
<hr>
<h2>添加图书</h2>
<form action="book?action=add" method="post">
图书编号:<input type="text" name="id"><br>
图书名称:<input type="text" name="name"><br>
作者:<input type="text" name="author"><br>
价格:<input type="text" name="price"><br>
<input type="submit" value="添加">
</form>
<hr>
<h2>删除图书</h2>
<form action="book?action=delete" method="post">
图书编号:<input type="text" name="id"><br>
<input type="submit" value="删除">
</form>
<hr>
<h2>修改图书</h2>
<form action="book?action=update" method="post">
图书编号:<input type="text" name="id"><br>
图书名称:<input type="text" name="name"><br>
作者:<input type="text" name="author"><br>
价格:<input type="text" name="price"><br>
<input type="submit" value="修改">
</form>
<hr>
<h2>查询图书</h2>
<form action="book?action=get" method="post">
图书编号:<input type="text" name="id"><br>
<input type="submit" value="查询">
</form>
<hr>
<h2>所有图书</h2>
<table border="1">
<tr>
<th>图书编号</th>
<th>图书名称</th>
<th>作者</th>
<th>价格</th>
</tr>
<c:forEach items="${bookList}" var="book">
<tr>
<td>${book.id}</td>
<td>${book.name}</td>
<td>${book.author}</td>
<td>${book.price}</td>
</tr>
</c:forEach>
</table>
</body>
</html>
```
阅读全文
相关推荐
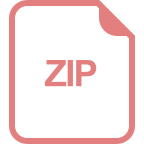
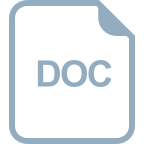
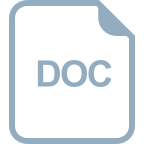
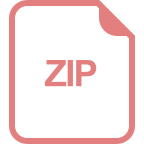
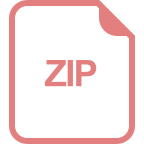
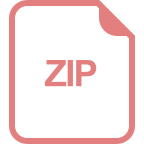
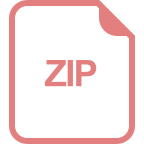
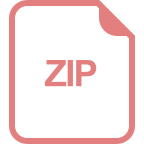
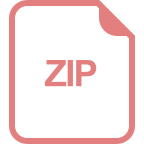
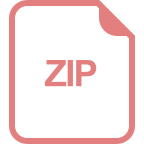
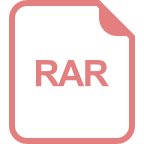
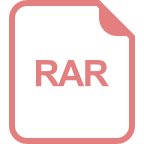