C#aspose.words如何获得单元格的高度
时间: 2024-12-11 20:30:36 浏览: 4
在Aspose.Words for .NET中,如果你想要获取文档表格中的单元格高度,你可以按照以下步骤操作:
1. 首先,你需要加载Word文档并定位到包含表格的部分:
```csharp
Document doc = new Document("your_document.docx");
Table table = doc.Tables[0]; // 假设你要处理的是第一个表格
```
2. 然后,访问单元格并获取其高度。Aspose.Words中的`TableCell`对象有一个属性`HeightInPoints`,它表示单元格的高度(单位是点),可以转换为你需要的其他单位(如毫米或英寸):
```csharp
float cellHeightInPoints = table.Cells[i].HeightInPoints;
float cellHeightInMillimeters = cellHeightInPoints * 72 / 28.35; // 将点转换为毫米(1点 = 1/72英寸)
```
这里`i`是你想要获取高度的那个单元格的索引。
注意:`HeightInPoints`返回的是精确的高度值,如果表格有行间距、段落间距等影响高度的因素,实际高度可能会有所不同。
相关问题
c#利用Aspose.Words向word表格内插入图片
要向Word表格内插入图片,你需要使用Aspose.Words库的Table类和Cell类。下面是一个示例代码,展示了如何在指定的表格单元格内插入图片:
```csharp
using Aspose.Words;
using System.Drawing;
class Program{
static void Main(string[] args)
{
// 加载Word文档
Document doc = new Document("input.docx");
// 获取要插入图片的表格
Table table = doc.GetChildNodes(NodeType.Table, true)[0] as Table;
// 获取表格的第一个单元格
Cell cell = table.Rows[0].Cells[0];
// 创建一个新的图片对象
Shape img = new Shape(doc, ShapeType.Image);
// 设置图片的位置和大小
img.Left = 0;
img.Top = 0;
img.Width = 100;
img.Height = 100;
// 加载要插入的图片
using (Image image = Image.FromFile("image.jpg"))
{
// 将图片添加到Shape对象中
img.ImageData.SetImage(image);
}
// 清空单元格内容
cell.RemoveAllChildren();
// 将图片插入到单元格中
cell.AppendChild(img);
// 保存文档
doc.Save("output.docx");
}
}
```
在上述代码中,首先加载了要编辑的Word文档(假设为"input.docx")。然后获取要插入图片的表格(这里假设你的文档中只有一个表格),以及要在其中插入图片的单元格。接下来,创建一个新的图片对象,并设置图片的位置和大小。然后加载要插入的图片,并将其添加到图片对象中。接着,清空单元格的内容,并将图片对象插入到单元格中。最后,保存文档(保存为"output.docx")。
请确保你已经将Aspose.Words库添加到你的项目中,并根据需要调整表格、单元格、图片的位置、大小和文件路径。
C# 在Aspose.Cells中插入由Byte[]转成的Image
在 Aspose.Cells 中,可以使用以下代码将 byte[] 转换为 Image 对象,并将其插入到 Excel 表格中:
```csharp
byte[] bytes = // 从某个地方获取 byte 数组
Image image;
using (MemoryStream ms = new MemoryStream(bytes))
{
image = Image.FromStream(ms);
}
// 插入图片到指定单元格
int rowIndex = // 指定行索引
int colIndex = // 指定列索引
int pictureIndex = worksheet.Pictures.Add(rowIndex, colIndex, image);
```
这里使用了 MemoryStream 将 byte[] 转换为流,然后使用 Image.FromStream 方法将流转换为 Image 对象。然后使用 Aspose.Cells.Worksheet.Pictures.Add 方法将图片插入到指定单元格,并返回该图片在 Excel 中的索引,可以用于进一步操作该图片。
需要注意的是,Aspose.Cells 需要引用 Aspose.Words.Drawing 命名空间,以便正确处理图片。
阅读全文
相关推荐
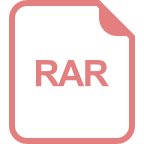
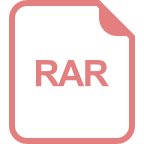
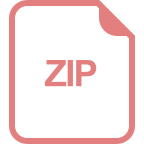
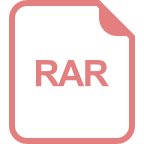
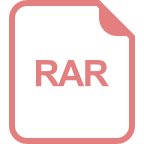
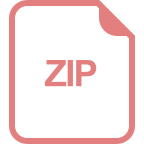
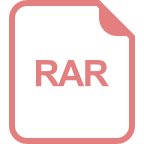
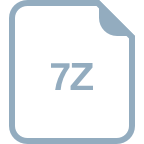
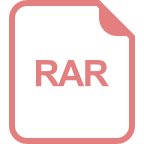
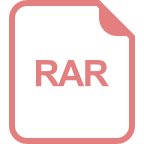
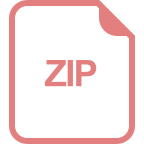
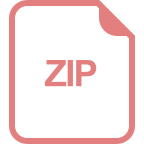
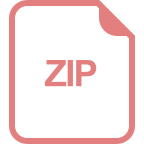
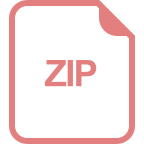

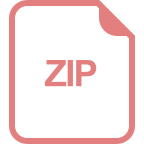