c语言 使用指针方法 定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年问题。
时间: 2024-12-18 12:32:25 浏览: 23
在C语言中,可以使用指针操作结构体变量并考虑闰年问题来计算某一天在这个年内是第几天。首先,我们需要定义一个包含年、月、日的结构体,并编写函数来处理闰年的判断。这里是一个简单的示例:
```c
#include <stdio.h>
// 结构体定义
typedef struct {
int year;
int month;
int day;
} Date;
// 判断是否为闰年的辅助函数
int is_leap_year(int year) {
if (year % 4 != 0)
return 0; // 非闰年,直接返回0
else if (year % 100 != 0)
return 1; // 年份能被4整除且不是世纪年,是闰年
else if (year % 400 != 0)
return 0; // 世纪年需满足同时被400整除才是闰年
else
return 1;
}
// 计算天数的函数,考虑闰年情况
int days_in_month(Date date) {
switch (date.month) {
case 1: case 3: case 5: case 7: case 8: case 10: case 12:
return 31; // 除2月外的非闰年月份都是31天
case 2: {
int days = is_leap_year(date.year) ? 29 : 28; // 2月有28天或29天
return days;
}
default:
return -1; // 输入错误,月份无效
}
}
// 主函数演示如何使用
int main() {
Date my_date = {2023, 2, 28}; // 设置日期
int total_days = 0;
for (int i = 1; i < my_date.month; ++i) {
if (is_leap_year(my_date.year))
total_days += days_in_month({my_date.year, i, 29});
else
total_days += days_in_month({my_date.year, i, 31});
}
total_days += my_date.day;
printf("The day %d/%d/%d is the %dth day of this year.\n",
my_date.day, my_date.month, my_date.year, total_days);
return 0;
}
```
在这个例子中,`days_in_month` 函数会根据输入的月份和是否为闰年来计算总天数。然后主函数中遍历到给定日期前的所有月份,累加天数,最后加上给定日期的天数即得到结果。
阅读全文
相关推荐





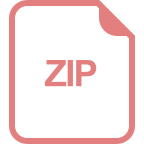








