python连连看游戏源代码
时间: 2024-10-06 18:02:15 浏览: 69
Python连连看游戏的源代码通常包含以下几个部分:
1. **主界面**:首先会有一个用户界面模块,比如Tkinter或Pygame库用于创建窗口、绘制棋盘、显示提示等。
```python
import pygame
board = [[0 for _ in range(size)] for _ in range(size)]
pygame.init()
screen = pygame.display.set_mode((size * cell_size, size * cell_size))
```
2. **游戏逻辑**:包括生成随机匹配的数字对、检查是否能消除一对、消除操作等函数。
```python
def create_pairs():
pairs = []
while len(pairs) < num_pairs:
x, y = random.randint(0, size - 1), random.randint(0, size - 1)
if board[x][y] == 0:
# 添加一对未连接的数字
pairs.append((x, y))
return pairs
def check_connection(x1, y1, x2, y2):
dx, dy = abs(x1 - x2), abs(y1 - y2)
return dx + dy <= 2
```
3. **事件处理**:监听用户的点击事件,并更新游戏状态。
```python
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONDOWN:
pos = pygame.mouse.get_pos()
row, col = pos // cell_size, pos % cell_size
if board[row][col] != 0:
# 用户尝试消除已经连接的点,忽略它
continue
connected_to = find_connected(row, col)
if connected_to:
# 更新棋盘并减少一对
update_board(row, col, connected_to)
```
4. **游戏结束条件**:当所有配对都被消除或达到最大步数限制时,游戏结束。
5. **循环和渲染**:在每次循环中,更新屏幕内容,直到用户关闭游戏窗口。
完整源码可能相当复杂,尤其是涉及到图形交互的部分,但以上是一个基础框架的概览。如果你想获取具体的源代码,可以在GitHub上搜索"Python Connect Four/Tic Tac Toe"找到示例项目。
阅读全文
相关推荐
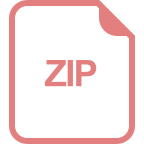
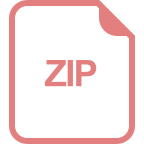
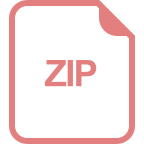















