C语言创建一个单链表并要求能够实现查找、插入、删除、打印基本操作,链表数据由键盘读入完整代码
时间: 2024-09-26 21:05:06 浏览: 10
在C语言中创建一个简单的单链表,包括查找、插入、删除和打印操作,我们需要定义链表结构体、相关的函数以及主程序来完成这些任务。下面是一个基础示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表为空
Node* createList() {
return NULL;
}
// 查找元素
Node* search(Node* head, int target) {
Node* current = head;
while (current != NULL) {
if (current->data == target)
return current;
current = current->next;
}
return NULL; // 如果未找到目标元素,则返回NULL
}
// 插入元素
void insert(Node** head, int value) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
// 删除元素
void delete(Node** head, int target) {
if (*head == NULL)
return;
if ((*head)->data == target) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != target) {
current = current->next;
}
if (current->next != NULL) {
Node* toDelete = current->next;
current->next = current->next->next;
free(toDelete);
}
}
// 打印链表
void printList(Node* head) {
while (head != NULL) {
printf("%d -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* head = createList();
// 输入数据并插入
int n, value;
printf("Enter number of elements: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("Enter element %d: ", i+1);
scanf("%d", &value);
insert(&head, value);
}
// 打印链表
printList(head);
// 用户输入查询
int target;
printf("Search for an element (press -1 to quit): ");
scanf("%d", &target);
while (target != -1) {
Node* found = search(head, target);
if (found != NULL) {
printf("Found element %d at position %ld.\n", target, (long)(found - head));
} else {
printf("Element not found in the list.\n");
}
printf("Search for an element (press -1 to quit): ");
scanf("%d", &target);
}
return 0;
}
```
相关推荐
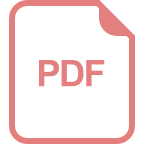
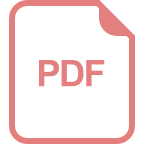
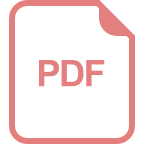














