如何在C#中实现一个界面,当显示包含确认和取消按钮的弹窗时,只有在用户停留并阅读至少5秒之后,确认按钮才解锁可点击?
时间: 2024-11-03 21:21:08 浏览: 8
在 C# 的 WPF 或 Windows Forms 中,你可以利用 `DispatcherTimer` 和 `Window` 的 `Closing` 事件来实现这种功能。以下是使用 Windows Forms 的示例代码:
```csharp
using System;
using System.Windows.Forms;
public partial class Form1 : Form
{
private readonly DispatcherTimer _timer;
private bool _canClickConfirm = false;
private DateTime _startReading;
public Form1()
{
InitializeComponent();
// 初始化计时器
_timer = new DispatcherTimer();
_timer.Interval = TimeSpan.FromSeconds(5); // 5秒间隔
_timer.Tick += Timer_Tick;
// 添加关闭窗口事件处理,防止用户直接关闭而未读完
this.Closing += Form_Closing;
}
private void Timer_Tick(object sender, EventArgs e)
{
if (!_isReading && DateTime.Now - _startReading >= _timer.Interval)
{
_canClickConfirm = true;
_timer.Stop();
// 在这里添加确认按钮的启用逻辑,例如:
btnConfirm.Enabled = _canClickConfirm;
}
}
private void btnConfirm_Click(object sender, EventArgs e)
{
if (_canClickConfirm)
{
// 用户阅读了至少5秒,现在可以点击确认
MessageBox.Show("确认按钮已解锁");
}
else
{
MessageBox.Show("请先阅读5秒钟!");
}
}
private void Form_Closing(object sender, CancelEventArgs e)
{
// 防止用户直接关闭而不满足阅读时间
if (!_canClickConfirm)
{
MessageBox.Show("请阅读至少5秒钟再关闭窗口。");
e.Cancel = true;
}
}
private void StartReading()
{
_isReading = true; // 假设有一个字段或属性_isReading用于跟踪是否正在阅读
_startReading = DateTime.Now;
}
}
// 在你需要展示对话框的地方调用StartReading()函数
private void ShowConfirmationDialog()
{
// 示例调用,假设form1实例已经存在
form1.StartReading();
form1.ShowDialog();
}
```
这段代码会在打开对话框时启动计时器,如果用户在5秒内尝试关闭窗口,会提示他们需要阅读更长时间。5秒后,确认按钮才会变为可用。用户点击确认按钮时,会检查是否有足够的阅读时间。
注意,实际应用中可能需要根据你的UI设计调整逻辑,例如替换 `_isReading` 和 `btnConfirm.Enabled` 的实现方式。
阅读全文
相关推荐
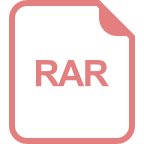
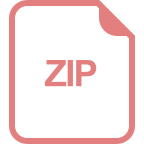
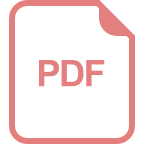
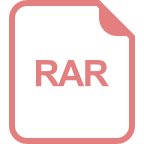
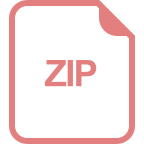
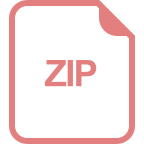
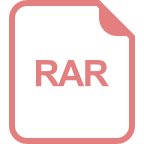
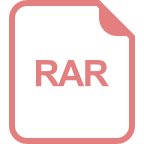
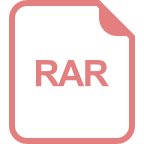
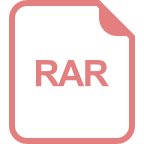
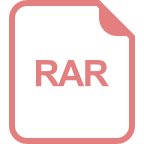
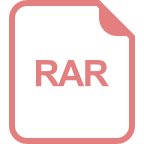
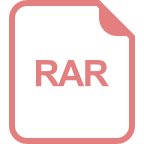
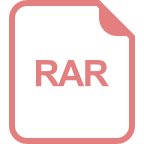
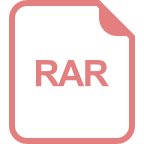
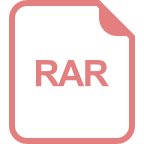
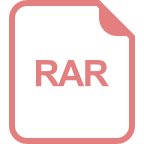