c语言将pdf转多页图片 windows
时间: 2024-01-31 17:03:56 浏览: 33
根据提供的引用内容,我们可以使用C语言中的Poppler库来将PDF转换为多页图片。具体步骤如下:
1.安装Poppler库。可以在官网下载源代码并编译安装,也可以使用包管理器进行安装。
2.在代码中引入Poppler库的头文件和相关的库文件。
3.使用Poppler库中的函数打开PDF文件。
4.使用Poppler库中的函数获取PDF文件的页数。
5.使用Poppler库中的函数将PDF文件的每一页转换为图片。
6.保存转换后的图片。
下面是一个简单的示例代码,将PDF文件转换为多页图片并保存在指定目录下:
```c
#include <poppler.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]) {
char *pdf_path = "example.pdf"; // PDF文件路径
char *img_dir = "example_images"; // 图片保存目录
int img_res = 150; // 图片分辨率
// 打开PDF文件
PopplerDocument *doc = poppler_document_new_from_file(pdf_path, NULL, NULL);
if (!doc) {
fprintf(stderr, "Failed to open PDF file: %s\n", pdf_path);
return 1;
}
// 获取PDF文件的页数
int num_pages = poppler_document_get_n_pages(doc);
// 创建图片保存目录
char *cmd = malloc(strlen(img_dir) + 20);
sprintf(cmd, "mkdir -p %s", img_dir);
system(cmd);
free(cmd);
// 将PDF文件的每一页转换为图片
for (int i = 0; i < num_pages; i++) {
PopplerPage *page = poppler_document_get_page(doc, i);
char *img_path = malloc(strlen(img_dir) + 20);
sprintf(img_path, "%s/page%d.png", img_dir, i + 1);
poppler_page_render_to_file(page, img_path, img_res, img_res, -1, 0);
free(img_path);
poppler_page_free(page);
}
// 关闭PDF文件
poppler_document_free(doc);
printf("PDF转换为图片成功!\n");
return 0;
}
```
相关推荐
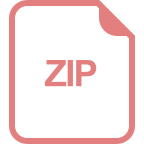
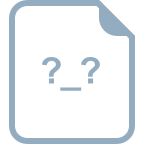
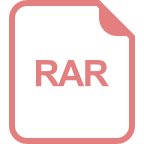














