AttributeError: 'numpy.float64' object has no attribute 'strip'怎么解决
时间: 2023-07-31 17:07:01 浏览: 285
这个错误是因为 `numpy.float64` 对象没有 `strip()` 方法。`strip()` 方法是字符串对象的方法,用于移除字符串开头和结尾的空格。如果你尝试将一个 `numpy.float64` 对象传递给 `strip()` 方法,就会出现该错误。
要解决这个问题,你可以将读取的数字转换为字符串类型,然后再应用 `strip()` 方法。以下是一个示例:
```python
import numpy as np
# 假设你有一个包含数字的 numpy 数组
numbers = np.array([1.0, 2.0, 3.0, 4.0, 5.0])
# 将数组中的数字转换为字符串类型,并使用逗号分隔
formatted_numbers = ', '.join(str(num) for num in numbers)
print(formatted_numbers)
```
在上述示例中,我们使用了列表推导式和 `join()` 方法,将 numpy 数组中的每个数字先转换为字符串,然后使用逗号连接起来。这样就避免了 `strip()` 方法使用在 `numpy.float64` 对象上引发错误的问题。
相关问题
AttributeError: 'numpy.float64' object has no attribute 'strip'解决
AttributeError: 'numpy.float64' object has no attribute 'strip'是一个常见的错误,通常出现在使用strip()函数对numpy中的float64类型数据进行字符串处理时。strip()函数是用来去除字符串首尾的空格或者指定字符的函数,但是它不能应用于float类型的数据。
解决方法有两种:
1. 在使用strip()函数时,先将float类型数据转换成字符串类型,如下所示:
str(np.float64_data).strip()
2. 使用numpy中的vectorize()函数对strip()函数进行向量化处理,如下所示:
np_vectorize = np.vectorize(lambda x: x.strip() if type(x) == str else x)
np_vectorize(np_data)
AttributeError: numpy.float64 object has no attribute append
这个错误提示意味着你试图在一个 numpy.float64 对象上调用 append 方法,但是该对象并没有这个方法。numpy.float64 是一个标量类型,它不支持 append 操作。如果你想要将多个 float64 值存储到一个数组中,可以使用 numpy 数组或 Python 列表。
下面是一个使用 numpy 数组的示例代码:
```python
import numpy as np
# 创建一个空的 float64 数组
arr = np.array([], dtype=np.float64)
# 向数组中添加元素
arr = np.append(arr, 1.0)
arr = np.append(arr, 2.0)
arr = np.append(arr, 3.0)
print(arr) # 输出 [1. 2. 3.]
```
阅读全文
相关推荐
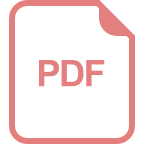
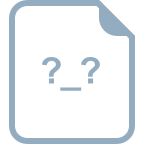
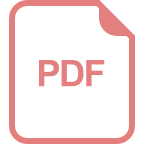













