如何在WPF中实现图片的自动轮播效果?
时间: 2024-10-01 21:07:27 浏览: 48
在WPF中实现图片的自动轮播效果,通常需要结合Timer和`ICollectionView`或`ICollectionViewBehavior`库。以下是简单的步骤:
1. **创建一个ICollectionView**:
- 在`ViewModel`中,使用`ICollectionView`来管理你的图片序列。初始化时加载所有图片,然后在`ICollectionView`上设置布局和分页。
```csharp
private ICollectionView _imageCollectionView;
public ICollectionView ImageCollectionView
{
get => _imageCollectionView ?? (_imageCollectionView = CollectionViewSource.GetDefaultView(Images));
set => _imageCollectionView = value;
}
private List<Uri> Images { get; } = LoadImages(); // 假设这是你的图片集合
```
2. **定时器和滚动索引**:
- 创建一个`System.Timers.Timer`实例,定期更改`ICollectionView`的当前页面,模拟轮播效果。比如每5秒滚动一次。
```csharp
private Timer _timer;
private int _currentIndex;
private async void StartAutoScroll()
{
_timer = new Timer(5000); // 每5秒
_timer.Elapsed += OnTimedEvent;
_timer.Start();
}
private async void StopAutoScroll()
{
_timer.Stop();
_timer.Elapsed -= OnTimedEvent;
}
private void OnTimedEvent(object sender, ElapsedEventArgs e)
{
await Task.Run(() =>
{
_currentIndex = (_currentIndex + 1) % Images.Count;
ImageCollectionView.MoveCurrentTo(Images[_currentIndex]);
});
}
```
3. **停止和暂停功能**:
- 提供一个方法来暂停或停止轮播,如上面的`StopAutoScroll()`。
4. **XAML绑定**:
- 将`ICollectionView`绑定到UI元素,例如使用`WrapPanel`包裹`Image`控件,使其在滚动时自动换位。
```xaml
<WrapPanel ItemsSource="{Binding ImageCollectionView}">
<WrapPanel.ItemsPanel>
<ItemsPanelTemplate>
<ItemsStackPanel Orientation="Horizontal"/>
</ItemsPanelTemplate>
</WrapPanel.ItemsPanel>
<Image Source="{Binding RelativeSource={RelativeSource AncestorType=WrapPanel}, Path=Items[CurrentPosition]}" />
</WrapPanel>
```
阅读全文
相关推荐
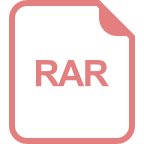
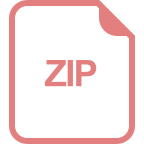
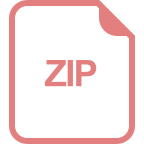
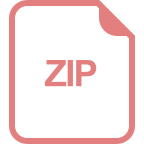
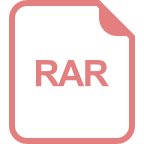
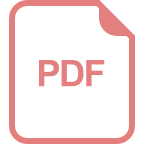
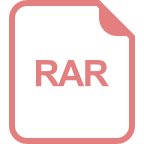
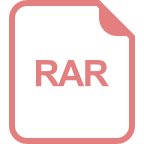
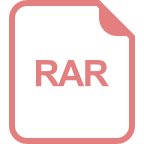
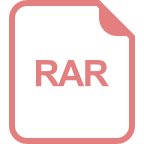
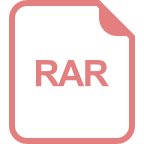
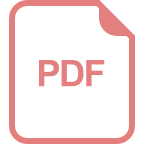
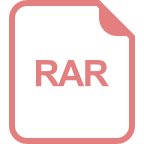
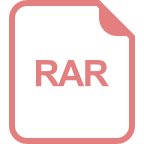
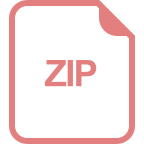
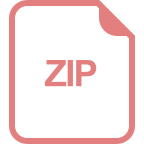
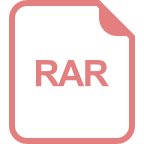
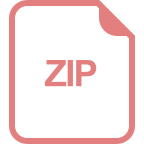
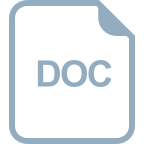