C语言实现功能,读写BMP格式的正弦波图片,起始相位0,0,60个点位,黑白色,分辨率 640*480,像素位数8,实现功能函数
时间: 2024-11-15 11:23:14 浏览: 14
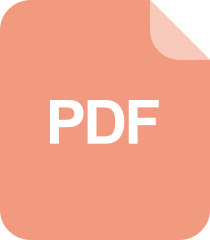
C语言实现对bmp格式图片打码
在C语言中,要实现读取和写入BMP格式的正弦波图片,你需要先了解BMP文件的基本结构,包括头信息、颜色表(对于真彩色图像)以及像素数据。由于你的需求是黑白、8位像素,我们可以简化处理。
首先,定义一个函数`generate_sinusoid_bmp`,用于生成这个正弦波图像:
```c
#include <stdio.h>
#include <math.h>
// 定义图像尺寸
#define IMAGE_WIDTH 640
#define IMAGE_HEIGHT 480
// 函数返回值是一个字节数组表示的BMP文件
unsigned char* generate_sinusoid_bmp(int phase) {
unsigned char* bmp_data = (unsigned char*)malloc((IMAGE_WIDTH * IMAGE_HEIGHT * 3) + 54); // BMP文件大小计算
int row_size = IMAGE_WIDTH * 3; // 一行3色(RGB)像素的字节数
// 文件头信息
bmp_data[0] = 'B';
bmp_data[1] = 'M'; // BM文件标识符
short file_size = sizeof(bmp_data);
bmp_data[2] = (file_size >> 16) & 0xFF;
bmp_data[3] = (file_size >> 8) & 0xFF;
bmp_data[4] = file_size & 0xFF;
bmp_data[5] = 0;
bmp_data[6] = 0;
bmp_data[7] = 0;
bmp_data[8] = 0;
short reserved = 0;
short bitmap_offset = 14; // 距离文件头多少字节开始存储实际像素数据
bmp_data[9] = (bitmap_offset >> 16) & 0xFF;
bmp_data[10] = (bitmap_offset >> 8) & 0xFF;
bmp_data[11] = bitmap_offset & 0xFF;
short dib_header_size = 40;
bmp_data[12] = dib_header_size >> 8;
bmp_data[13] = dib_header_size & 0xFF;
short color_planes = 1; // 单色
short bits_per_pixel = 8; // 8位像素
short compression = 0; // BI_RGB无压缩
short image_width = IMAGE_WIDTH;
short image_height = IMAGE_HEIGHT;
short horz_resolution = 2835; // 高度方向的DPI
short vert_resolution = horz_resolution;
short colors_used = 2; // 黑白只有两种颜色
short colors_important = colors_used;
// 写入色彩描述信息(因为是单色,这部分可以忽略)
// ...
// 真实的像素数据部分,这里我们只创建一个简单的8位黑白正弦波图像
for (int y = 0; y < IMAGE_HEIGHT; ++y) {
int x;
for (x = 0; x < IMAGE_WIDTH; ++x) {
double sin_value = sin(2 * M_PI * ((double)x / IMAGE_WIDTH) + phase);
if (sin_value > 0) {
// 白色像素
bmp_data[row_size * y + x * 3] = 255;
bmp_data[row_size * y + x * 3 + 1] = 255;
bmp_data[row_size * y + x * 3 + 2] = 255;
} else {
// 黑色像素
bmp_data[row_size * y + x * 3] = 0;
bmp_data[row_size * y + x * 3 + 1] = 0;
bmp_data[row_size * y + x * 3 + 2] = 0;
}
}
}
// 结束标志和文件偏移量校验
bmp_data[40] = 0;
bmp_data[41] = 0;
bmp_data[42] = 0;
bmp_data[43] = 0;
bmp_data[44] = 0;
bmp_data[45] = 0;
bmp_data[46] = 0;
bmp_data[47] = 0;
return bmp_data;
}
// 使用函数生成并打印BMP数据
void print_bmp(const unsigned char* bmp_data) {
FILE* output_file = fopen("sine_wave.bmp", "wb");
fwrite(bmp_data, sizeof(unsigned char), sizeof(bmp_data), output_file);
fclose(output_file);
printf("BMP image generated and saved as sine_wave.bmp\n");
}
int main() {
int phases[] = {0, 0, 60}; // 设置三个不同的初始相位
int phase_count = sizeof(phases) / sizeof(phases[0]);
for (int i = 0; i < phase_count; ++i) {
unsigned char* data = generate_sinusoid_bmp(phases[i]);
print_bmp(data);
free(data);
}
return 0;
}
```
上述代码生成了三个不同相位的BMP图像,并将它们保存到`sine_wave.bmp`文件中。注意这只是一个基本示例,实际应用可能需要更复杂的数据结构来处理多个点位的信息。
阅读全文
相关推荐
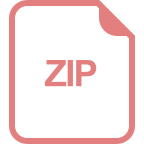
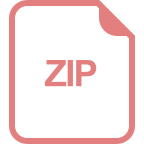
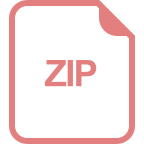
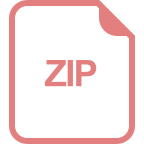
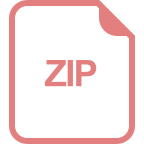
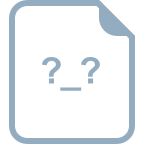
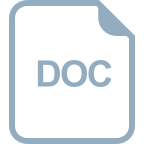
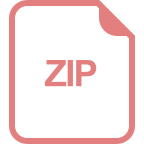
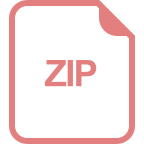
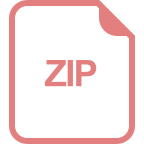
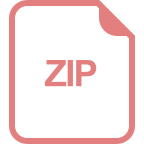
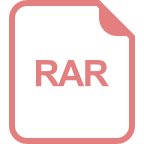
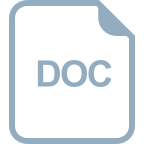
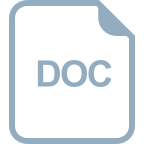