多线程和并发编程技术
时间: 2024-08-04 20:01:03 浏览: 78
并发编程和多线程是计算机科学中的关键概念,它们允许程序在同一时间执行多个任务,提高资源利用率。在Python中,可以利用`threading`模块来实现多线程:
1. **创建新线程**[^4]:
```python
import threading
def worker_function(name):
"""线程要执行的任务"""
print(f"Thread {name} started")
# 这里放置实际的工作代码
print(f"Thread {name} finished")
threads = []
for i in range(5): # 创建5个线程
thread = threading.Thread(target=worker_function, args=(i,))
threads.append(thread)
thread.start() # 启动线程
for thread in threads:
thread.join() # 等待所有线程完成
```
2. **共享数据和同步**[^4]:
- 使用`threading.Lock()`或其他锁机制(如`RLock`)保护共享资源,防止竞态条件(race condition)。
- `queue.Queue`或`concurrent.futures`模块的`ThreadPoolExecutor`用于在不同线程之间传递数据。
3. **进程与线程的区别**[^4]:
- 进程是独立的内存空间,每个进程有自己的资源副本;而线程共享同一进程的内存,因此更改会影响其他线程。
- 多进程间的通信通常通过文件、管道或消息队列(如`multiprocessing.Queue`),而线程间直接使用全局变量或特定的数据结构。
相关问题--
1. Python中如何避免多线程竞争条件?
2. 如何在Python中控制线程的数量以优化性能?
3. Python的`asyncio`库是如何实现异步非阻塞I/O的?
阅读全文
相关推荐
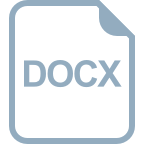
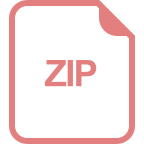
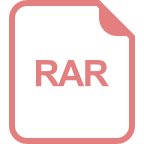
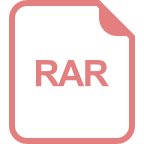
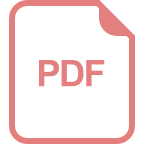
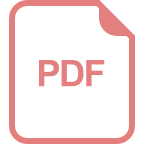
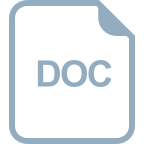
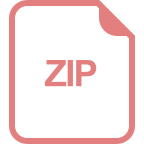
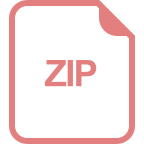
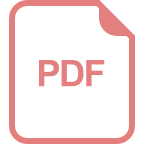
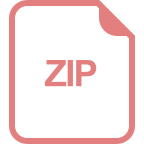
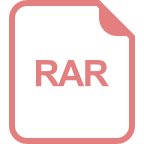
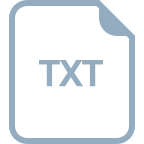
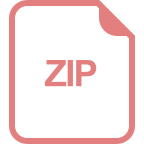
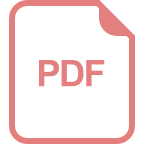
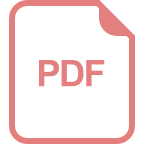
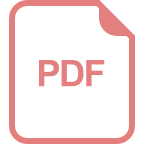