QT中用qcustomplot类写一个三角波
时间: 2024-06-20 22:00:22 浏览: 128
在Qt中使用`QCustomPlot`类创建一个三角波图形,你需要先确保已安装并包含了`QCustomPlot`库。以下是一个简单的步骤来创建一个三角波图表:
1. 引入必要的库:
```cpp
#include <QApplication>
#include <QCustomPlot>
#include <QPainter>
#include <QPen>
```
2. 创建`QCustomPlot`对象:
```cpp
QCustomPlot *chart = new QCustomPlot;
```
3. 设置绘图区域:
```cpp
chart->setCanvasColor(Qt::white);
chart->xAxis->setLabel("Time (s)");
chart->yAxis->setLabel("Amplitude");
```
4. 定义三角波函数:
```cpp
double triangleWave(double t, double freq = 1.0, double peak = 1.0) {
return peak * std::abs(2 * std::sin(2 * M_PI * freq * t));
}
```
5. 添加数据系列(在这一步骤中,我们将在0到1秒的时间间隔上生成100个点的三角波):
```cpp
int numPoints = 100;
for (int i = 0; i <= numPoints; ++i) {
double xValue = static_cast<double>(i) / numPoints;
double yValue = triangleWave(xValue);
chart->addScatterSeries(xValue, yValue);
}
```
6. 绘制三角波:
```cpp
chart->replot();
```
7. 显示图形:
```cpp
QWidget *parent = new QWidget;
parent->setLayout(new QVBoxLayout {chart});
parent->show();
```
8. 运行应用:
```cpp
QApplication app(argc, argv);
return app.exec();
```
阅读全文
相关推荐

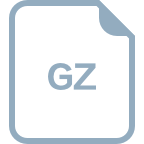












