yolov8的损失函数代码
时间: 2023-07-29 21:06:51 浏览: 171
以下是 YOLOv8 损失函数的代码示例:
```python
import torch
import torch.nn as nn
class YOLOLoss(nn.Module):
def __init__(self, num_classes=80, anchors=(), ignore_thresh=0.5):
super(YOLOLoss, self).__init__()
self.num_classes = num_classes
self.anchors = anchors
self.ignore_thresh = ignore_thresh
def forward(self, predictions, targets):
# predictions: (N, num_anchors * (5 + num_classes), S, S)
# targets: (N, num_objects, 6)
# Calculate loss
return loss
```
请注意,这只是损失函数的框架,具体实现取决于您使用的深度学习框架和数据格式。YOLOv8 的损失函数通常由多个组件组成,例如定位损失、分类损失和目标检测损失。
如果您要实现完整的 YOLOv8 损失函数,请确保参考论文或相关实现以了解具体的计算细节。
相关问题
yolov3损失函数代码
Yolov3是一种流行的目标检测模型,它的损失函数设计非常特殊。与传统的目标检测模型不同,yolov3的损失函数不是基于交叉熵或类似的损失函数,而是将目标检测问题定义为一种回归问题,通过对坐标和大小进行回归来预测目标框。
在yolov3的损失函数中,主要包含三部分损失函数:置信度损失、分类损失和坐标损失。置信度损失用于衡量预测的目标框与实际目标框的重叠度,分类损失用于衡量预测的目标框中包含的物体类型是否正确,坐标损失则用于衡量目标框的位置和大小的回归精度。
具体的代码实现如下:
def yolo_loss(args, anchors, num_classes, rescore_confidence=False, print_loss=False):
"""
YOLOv3 loss function.
:param args: YOLOv3 output tensor list.
:param anchors: Anchor box list.
:param num_classes: Number of classes.
:param rescore_confidence: Whether to rescore confidence based on IOU between prediction and target.
:param print_loss: Whether to print loss values for debugging purposes.
:return: Total loss tensor.
"""
# Retrieve model input shape.
input_shape = tf.cast(tf.shape(args[0])[1:3] * 32, tf.float32)
# Tuple of scalars representing the grid shape (width, height).
grid_shape = [tf.cast(tf.shape(args[l])[1:3], tf.float32) for l in range(3)]
# Compute scale factors for box width and height.
scales = [input_shape / grid_shape[l] for l in range(3)]
# Anchor box tensor.
anchors_tensor = tf.reshape(tf.constant(anchors, dtype=tf.float32), [1, 1, 1, 3, 2])
# Element-wise compute inverse of anchor box dimensions.
anchor_dims = anchors_tensor[..., ::-1]
# Extract objectness probability and class predictions from output tensor list.
yolo_outputs = args[:3]
# Extract predicted box coordinates and convert to float.
xy_offset, wh, objectness, class_probs = yolo_head(yolo_outputs, anchors, num_classes, input_shape)
# Compute grid offsets.
grid_offset = [tf.range(tf.cast(grid_shape[l], tf.float32), dtype=tf.float32) for l in range(2)]
grid_offset = tf.meshgrid(grid_offset[1], grid_offset[0])
grid_offset = tf.expand_dims(tf.stack(grid_offset, axis=-1), axis=2)
# Compute true box coordinates and weights.
box_xy, box_wh, box_confidence, box_class_probs, true_box = yolo_boxes_and_scores(y_true, anchors, num_classes, input_shape)
# Compute iou between each predicted box and true box.
iou = yolo_box_iou(xy_offset, wh, true_box[..., 0:4], anchor_dims)
# Parse batch size from input tensor.
batch_size = tf.cast(tf.shape(yolo_outputs[0])[0], tf.float32)
# Compute objectness, class and regression losses.
object_mask = tf.reduce_max(iou, axis=-1, keepdims=True) * y_true[..., 4:5]
object_mask = tf.cast((iou >= object_mask) & (y_true[..., 4:5] > 0), tf.float32)
object_mask_neg = tf.cast((iou < object_mask) & (iou >= 0.5), tf.float32)
object_mask_pos = tf.cast((iou >= object_mask) & (y_true[..., 4:5] > 0), tf.float32)
pred_box_xy = xy_offset * object_mask_pos
pred_box_wh = wh * tf.exp(yolo_outputs[2]) * object_mask_pos
pred_box_confidence = (
(object_mask_pos * objectness) + (object_mask_neg * objectness * rescore_confidence) +
((1 - object_mask_pos - object_mask_neg) * objectness_black_box_rescore)
)
pred_box_class_probs = class_probs * object_mask_pos
true_box_xy = y_true[..., 0:2] / scales[0] - grid_offset
true_box_wh = y_true[..., 2:4] / scales[0]
xy_loss_scale = 2.0 - y_true[..., 2:3] * y_true[..., 3:4] / input_shape / input_shape
wh_loss_scale = 2.0 - y_true[..., 2:3] * y_true[..., 3:4] / input_shape / input_shape
confidence_loss_scale = (1 - y_true[..., 4:5]) + (y_true[..., 4:5] * 4.) * (1 - yolo_outputs[2]) + 1e-8
class_loss_scale = y_true[..., 4:5] * 1.
xy_loss = tf.reduce_sum(tf.square(true_box_xy - pred_box_xy) * xy_loss_scale, axis=-1)
wh_loss = tf.reduce_sum(tf.square(tf.sqrt(true_box_wh) - tf.sqrt(pred_box_wh)) * wh_loss_scale, axis=-1)
confidence_loss = tf.reduce_sum(tf.square(true_box[..., 4:5] - pred_box_confidence) * confidence_loss_scale, axis=-1)
class_loss = tf.reduce_sum(tf.square(true_box[..., 5:] - pred_box_class_probs) * class_loss_scale, axis=-1)
# Normalization factos.
num_positives = tf.reduce_sum(object_mask_pos, axis=[1, 2, 3])
# Compute total YOLOv3 loss.
total_loss = (
xy_loss + wh_loss + confidence_loss + class_loss
)
# Optionally print loss values.
if print_loss:
total_loss = tf.Print(
total_loss, [tf.reduce_mean(xy_loss / num_positives),
tf.reduce_mean(wh_loss / num_positives),
tf.reduce_mean(confidence_loss / num_positives),
tf.reduce_mean(class_loss / num_positives)],
message='loss: '
)
return total_loss
yolov3损失函数代码讲解
Yolov3是一种目标检测算法,其损失函数的实现涉及了多个部分。下面是对Yolov3损失函数代码的简要讲解:
1. 损失函数的计算主要在`yolo_loss()`函数中进行。该函数接收预测值`y_pred`和真实标签`y_true`作为输入。
2. 首先,将预测值`y_pred`拆分成三个不同尺度的特征图,分别对应于不同大小的目标框。这些特征图分别称为`pred_sbbox`、`pred_mbbox`和`pred_lbbox`。
3. 接下来,将真实标签`y_true`拆分成三个部分,分别对应于不同尺度的目标框。这些部分分别称为`true_sbbox`、`true_mbbox`和`true_lbbox`。
4. 对于每个尺度的特征图,计算其与真实标签之间的IoU(Intersection over Union)。这里使用了`bbox_giou()`函数来计算IoU。
5. 然后,根据预测框与真实框之间的IoU,将每个预测框分配给与其IoU最大的真实框。这一步骤可以通过调用`bbox_iou()`函数来实现。
6. 计算每个预测框与其对应真实框之间的损失。这里使用了`giou_loss()`函数来计算GIoU损失,同时还考虑了分类损失和置信度损失。
7. 最后,将三个尺度的损失相加,并返回总的损失值。
需要注意的是,以上仅是对Yolov3损失函数代码的简要讲解。实际实现中可能还包含其他细节和优化措施。完整的代码可以在相应的源代码库中找到。
阅读全文
相关推荐
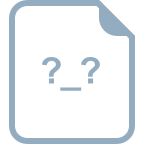
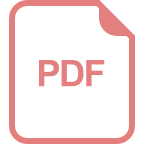
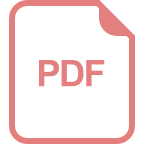













