能否提供一个关于如何在PyCharm中应用A*算法解决旅行商问题(TSP)的路径规划问题的具体步骤和示例代码?
时间: 2024-12-24 16:21:29 浏览: 0
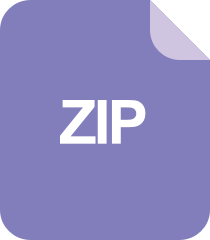
基于 Pycharm 和 QGIS 进行插件开发,解决旅行商问题(TSP)(Python)
在PyCharm中应用A*算法解决旅行商问题(TSP,Traveling Salesman Problem)需要一些步骤,包括设置问题、实现搜索算法和优化路径。这里是一个简化的例子,并非完整的项目,但可以帮助理解基本流程:
1. **设置环境**:
首先,安装必要的库,如`numpy`, `pandas`, 和`matplotlib`,如果还没安装,可以使用`pip install numpy pandas matplotlib`。
2. **创建图数据结构**:
创建一个表示城市之间距离的邻接矩阵或邻接列表。例如,你可以创建一个二维数组,其中`matrix[i][j]`表示从城市i到城市j的距离。
```python
import numpy as np
# 示例数据(假设4个城市)
cities = ['A', 'B', 'C', 'D']
distances = np.array([[0, 5, 10, 8],
[5, 0, 6, 7],
[10, 6, 0, 9],
[8, 7, 9, 0]])
```
3. **A*算法核心**:
实现A*算法的核心部分,包括计算启发式函数(估计从当前节点到目标节点的最短距离),评估函数(总代价加上启发式代价),以及选择下一个最佳节点。
```python
def heuristic(a, b):
return distances[a][b]
def astar(start, end, graph, closed_set=None, open_set=None):
if open_set is None:
open_set = [(0, start)]
if closed_set is None:
closed_set = set()
g_scores = {start: 0}
f_scores = {start: heuristic(start, end)}
while open_set:
current = min(open_set, key=lambda x: f_scores[x[1]])
if current[1] == end:
path = reconstruct_path(current[1], closed_set)
return path, g_scores[end]
open_set.remove(current)
closed_set.add(current[1])
for neighbor in get_neighbors(graph, current[1]):
tentative_g_score = g_scores[current[1]] + graph[current[1]][neighbor]
if neighbor not in closed_set or tentative_g_score < g_scores.get(neighbor, float('inf')):
if neighbor not in open_set or tentative_g_score < f_scores[neighbor]:
g_scores[neighbor] = tentative_g_score
f_scores[neighbor] = tentative_g_score + heuristic(neighbor, end)
open_set.append((f_scores[neighbor], neighbor))
return None, None # No solution found
def get_neighbors(graph, node):
return [n for n in range(len(graph)) if graph[node][n] > 0]
def reconstruct_path(end, closed_set):
path = [end]
parent = {end: None}
while path[-1] != 'A':
parent[path[-1]] = path[-2]
next_node = parent[path[-1]]
path.append(next_node)
path.reverse()
return path
```
4. **运行算法并可视化结果**:
完成算法后,使用`astar`函数找出最短路径,并绘制出来。
```python
solution, distance = astar('A', 'D', distances)
print(f"Solution: {solution}, Total Distance: {distance}")
plot_solution(cities, distances, solution)
```
阅读全文
相关推荐
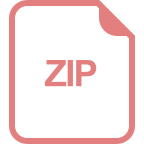
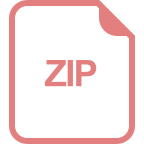


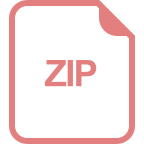
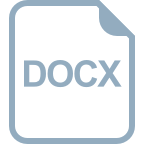
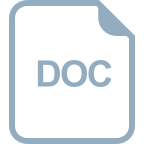
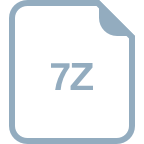
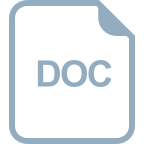
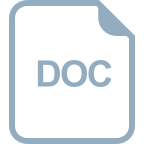
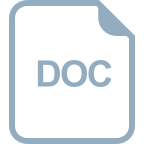
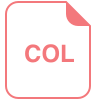
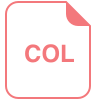
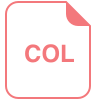
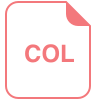
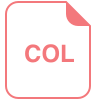
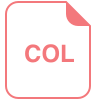