在一种运动控制模块的数据处理方法中怎么使用c++从运动指令打包线程中获取运动指令命令
时间: 2024-12-11 14:31:15 浏览: 7
在C++中,为了从运动指令打包线程中获取运动指令命令,你可以采取以下步骤:
1. **线程设计**:首先,创建一个单独的线程,这个线程负责监听运动指令并将其添加到一个共享数据结构(如队列、条件变量或者共享内存区域)中。可以使用`std::thread`库或者第三方线程库(如C++17后的`std::async`)来启动这个线程。
```cpp
#include <thread>
#include <queue>
class InstructionThread {
public:
void run() {
// 模拟接收到运动指令的逻辑
while (true) {
std::unique_lock<std::mutex> lock(mutex);
if (!instructions.empty()) { // 如果有新的指令
instruction = instructions.front();
instructions.pop(); // 移除并获取新指令
lock.unlock(); // 解锁,允许主线程访问
notify.notify_one(); // 醒醒主线程
break; // 如果只有一个指令,则退出循环
}
}
}
private:
std::queue<Command> instructions;
Command instruction;
std::mutex mutex;
std::condition_variable notify;
};
```
2. **主线程等待**:在主线程中,你需要创建一个线程安全的方式来接收指令。这通常涉及创建一个互斥量(mutex),条件变量(condition_variable),并阻塞在一个条件上,直到指令可用。
```cpp
InstructionThread thread;
thread.start();
// 在主线程中接收指令
std::unique_lock<std::mutex> lock(thread.mutex);
while (true) {
thread.notify.wait(lock, []{return !thread.instructions.empty();}); // 等待直到有新指令
if (!thread.instructions.empty()) {
Command received_command = thread.instruction; // 获取指令
// 进行指令处理...
}
}
```
3. **停止和销毁**:当不再需要运动指令时,记得在主线程中发送信号通知停止,然后关闭线程。
```cpp
void stop_thread() {
thread.instructions.push(nullptr); // 或者设置一个终止标识
notify.notify_all(); // 触发所有等待的线程
thread.join(); // 等待线程结束
}
// 在适当的时候调用stop_thread()
```
阅读全文
相关推荐
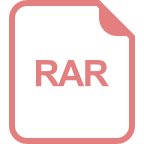
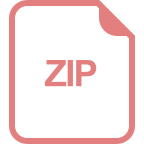
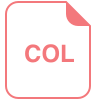
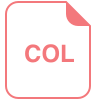
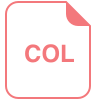
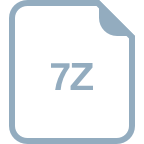
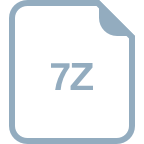
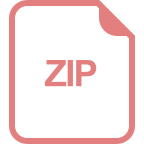
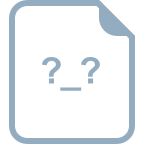
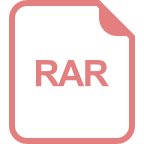
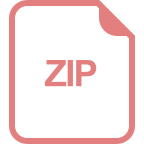
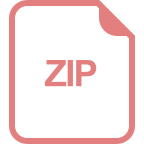
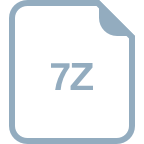
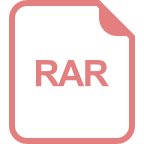
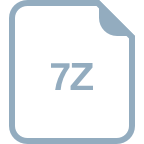