如何在react脚手架中实现一个评论区页面的制作jsx
时间: 2024-10-15 21:19:37 浏览: 25
在React脚手架(如Create React App)中创建一个评论区页面,你可以按照以下步骤来实现:
1. **设置项目结构**:
创建一个新的组件文件,例如`CommentSection.js`,这是存放评论区域逻辑的地方。
```jsx
// CommentSection.js
import React, { useState } from 'react';
const CommentSection = () => {
// 简单起见,这里我们仅展示状态管理
const [comments, setComments] = useState([]);
const [newComment, setNewComment] = useState('');
// 添加新评论函数
const addComment = (e) => {
e.preventDefault();
if (newComment.trim()) {
setComments([...comments, newComment]);
setNewComment('');
}
};
return (
<div>
{/* 显示已有评论 */}
<ul>
{comments.map((comment, index) => (
<li key={index}>{comment}</li>
))}
</ul>
{/* 新建评论表单 */}
<form onSubmit={addComment}>
<input
type="text"
value={newComment}
onChange={(e) => setNewComment(e.target.value)}
placeholder="请输入评论..."
/>
<button type="submit">提交</button>
</form>
</div>
);
};
export default CommentSection;
```
2. **导入并使用组件**:
在需要显示评论区的页面,如`App.js`或其他组件内,引入并使用这个`CommentSection`组件。
```jsx
// App.js 或者你想使用的组件
import React from 'react';
import CommentSection from './CommentSection';
function App() {
return (
<div className="App">
{/* 其他内容... */}
<CommentSection />
</div>
);
}
export default App;
```
3. **样式与交互**:
使用CSS来美化评论列表和表单,以及添加适当的事件处理和交互效果(比如删除按钮、加载更多等)。这取决于实际需求。
阅读全文
相关推荐
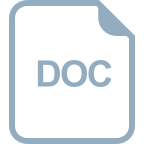
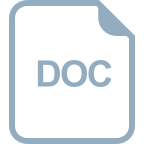
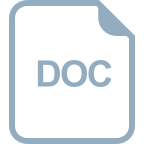
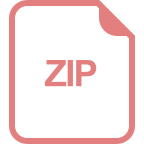
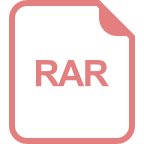
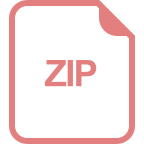
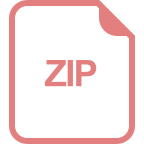
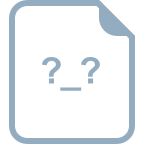
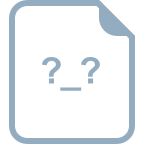
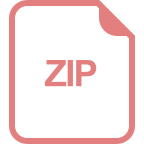
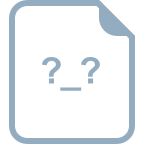
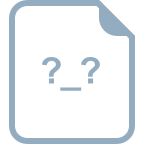
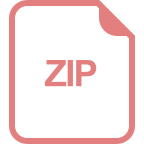
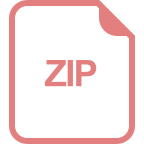
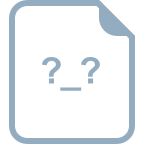
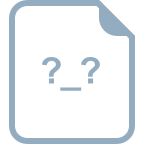
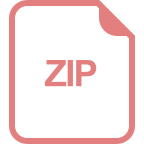
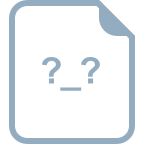