python遗传算法特征选择
时间: 2023-08-15 07:15:28 浏览: 91
遗传算法是一种优化算法,可以用于特征选择。在特征选择问题中,我们希望从给定的特征集合中选择出最具有代表性的特征子集,以提高机器学习模型的性能和效果。
下面是一种用遗传算法进行特征选择的一般步骤:
1. 初始化种群:随机生成一组特征子集,称为个体,作为初始种群。
2. 评估适应度:使用某种评价指标(如分类准确率、回归误差等)对每个个体进行适应度评估。
3. 选择操作:根据适应度值,选择一些个体作为父代,选择的方式可以是轮盘赌选择、锦标赛选择等。
4. 交叉操作:对选出的父代个体进行染色体交叉操作,生成新的后代个体。
5. 变异操作:对后代个体进行染色体变异操作,引入新的特征。
6. 评估适应度:对变异后的后代个体进行适应度评估。
7. 父代与后代合并:将父代和后代个体合并形成新的种群。
8. 重复步骤3至7,直到满足终止条件(例如达到最大迭代次数或找到最优特征子集)。
9. 返回最优特征子集作为最终结果。
需要注意的是,遗传算法的性能和效果与参数设置、适应度函数的选择以及交叉和变异操作的设计密切相关。因此,在使用遗传算法进行特征选择时,需要根据具体问题进行调整和优化,以获得最佳结果。
相关问题
python遗传算法特征选择代码实例
遗传算法是一种通过模拟生物进化过程来解决优化问题的算法。特征选择是机器学习中的一个重要任务,它可以帮助我们从大量的特征中选择出对目标变量具有最大预测能力的特征子集。下面是一个使用遗传算法进行特征选择的Python代码示例:
```python
import numpy as np
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# 创建一个示例数据集
X, y = make_classification(n_samples=100, n_features=10, random_state=42)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义适应度函数
def fitness_function(individual, X, y):
selected_features = X[:, individual]
clf = LogisticRegression()
clf.fit(selected_features, y)
return clf.score(selected_features, y)
# 定义遗传算法相关操作
def initialize_population(population_size, chromosome_length):
return np.random.randint(2, size=(population_size, chromosome_length))
def crossover(parent1, parent2):
crossover_point = np.random.randint(1, len(parent1)-1)
child1 = np.concatenate((parent1[:crossover_point], parent2[crossover_point:]))
child2 = np.concatenate((parent2[:crossover_point], parent1[crossover_point:]))
return child1, child2
def mutation(individual, mutation_rate):
for i in range(len(individual)):
if np.random.rand() < mutation_rate:
individual[i] = 1 - individual[i]
return individual
def selection(population, fitness_values):
sorted_indices = np.argsort(fitness_values)[::-1]
return population[sorted_indices][:len(population)//2]
# 设置遗传算法参数
population_size = 100
chromosome_length = X_train.shape[1]
mutation_rate = 0.01
num_generations = 50
# 初始化种群
population = initialize_population(population_size, chromosome_length)
# 进化过程
for generation in range(num_generations):
# 计算适应度值
fitness_values = np.array([fitness_function(individual, X_train, y_train) for individual in population])
# 选择种群中的优秀个体
selected_population = selection(population, fitness_values)
# 生成下一代种群
new_population = []
while len(new_population) < population_size:
parent1, parent2 = np.random.choice(selected_population, size=2, replace=False)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1, mutation_rate)
child2 = mutation(child2, mutation_rate)
new_population.append(child1)
new_population.append(child2)
population = np.array(new_population)
# 在测试集上进行评估
best_individual = population[np.argmax(fitness_values)]
selected_features = X_train[:, best_individual]
clf = LogisticRegression()
clf.fit(selected_features, y_train)
test_selected_features = X_test[:, best_individual]
accuracy = clf.score(test_selected_features, y_test)
print("Selected features accuracy:", accuracy)
```
这段代码演示了如何使用遗传算法进行特征选择。首先,它创建一个示例数据集,然后划分为训练集和测试集。接下来定义了适应度函数,该函数计算选定特征子集在逻辑回归模型上的准确度作为适应度值。然后,定义了遗传算法的各种操作,包括初始化种群、交叉、变异和选择。最后,通过迭代多个代进行进化,选择最佳个体,并在测试集上评估所选特征子集的准确度。
python 遗传算法 特征选择 开源_遗传算法之特征选择的python实现
遗传算法在特征选择中的应用是非常广泛的。以下是一个基于Python实现的遗传算法特征选择的示例代码:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
# 加载数据集
iris = load_iris()
X, y = iris.data, iris.target
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义适应度函数
def fitness(features):
X_train_selected = X_train[:, features]
X_test_selected = X_test[:, features]
clf = LogisticRegression()
clf.fit(X_train_selected, y_train)
score = clf.score(X_test_selected, y_test)
return score
# 遗传算法
def genetic_algorithm(size, gensize, retain, random_select, mutate):
population = []
for i in range(size):
chromosome = np.ones(gensize)
chromosome[:int(0.5*gensize)] = 0
np.random.shuffle(chromosome)
population.append(chromosome)
for i in range(100):
scores = []
for chromosome in population:
score = fitness(np.where(chromosome == 1)[0])
scores.append((score, chromosome))
scores.sort(reverse=True)
ranked_chromosomes = [x[1] for x in scores]
population = ranked_chromosomes[:retain]
while len(population) < size:
if np.random.rand() < random_select:
population.append(ranked_chromosomes[np.random.randint(0, retain)])
else:
chromosome1 = ranked_chromosomes[np.random.randint(0, retain)]
chromosome2 = ranked_chromosomes[np.random.randint(0, retain)]
new_chromosome = chromosome1.copy()
mask = np.random.rand(len(new_chromosome)) < 0.5
new_chromosome[mask] = chromosome2[mask]
if np.random.rand() < mutate:
mask = np.random.rand(len(new_chromosome)) < 0.5
new_chromosome[mask] = np.logical_not(new_chromosome[mask])
population.append(new_chromosome)
return np.where(scores[0][1] == 1)[0]
# 运行遗传算法
selected_features = genetic_algorithm(size=100, gensize=X.shape[1], retain=20, random_select=0.05, mutate=0.01)
print(selected_features)
```
在上面的代码中,我们使用逻辑回归作为分类器,定义一个适应度函数来评估每个个体(即特征子集)的好坏程度。遗传算法的主要过程包括初始化种群、评估适应度、选择、交叉和变异。最终输出的是被选择的特征的索引。
相关推荐
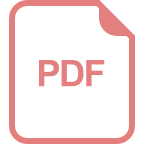
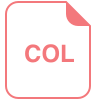
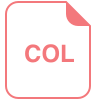
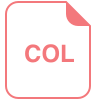
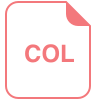
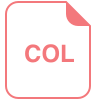







