Python自动化运维系统性能监控代码
时间: 2024-06-14 18:03:01 浏览: 16
Python在自动化运维中常用于性能监控,因为它提供了丰富的库和易于编程的特性。要编写一个基本的性能监控代码,通常会用到像`psutil`、`celery`(用于分布式任务)、`requests`(处理HTTP请求)和`logging`(日志记录)等库。以下是一个简化的示例,展示了如何使用这些工具来监控CPU、内存、网络和HTTP服务的性能:
```python
import psutil
import requests
from celery import Celery
import logging
# 初始化日志
logging.basicConfig(filename='app.log', level=logging.INFO)
# 创建Celery实例
app = Celery('tasks', broker='pyamqp://guest@localhost//')
@app.task
def monitor_service(url):
try:
response = requests.get(url, timeout=5)
response.raise_for_status()
logging.info(f"Service status: {response.status_code}")
except requests.exceptions.RequestException as e:
logging.error(f"Performance issue: {e}")
# 监控函数
def monitor_system():
cpu_percent = psutil.cpu_percent(interval=1)
memory_info = psutil.virtual_memory()
mem_percent = memory_info.percent
network_info = psutil.net_io_counters()
logging.info(f"CPU usage: {cpu_percent}%")
logging.info(f"Memory usage: {mem_percent}%")
logging.info(f"Network traffic: {network_info.bytes_sent / (1024 * 1024)} MB sent")
# 每隔一定时间监控一次服务
app.send_task('monitor_service', args=['http://your-service.com'])
if __name__ == "__main__":
monitor_system()
```
相关推荐
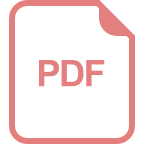
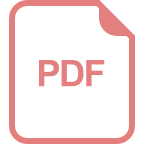
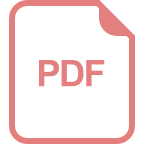














