如何使用C++编程语言分别计算并统计一段文本中包含的数字字符数量、字母字符数量以及其它字符(非数字和字母)的数量?
时间: 2024-10-21 15:03:20 浏览: 37
在C++中,你可以使用`<cctype>`库中的成员函数来区分字符类型,并使用`<string>`库操作字符串。下面是一个简单的步骤来计算和统计这些信息:
1. 定义一个函数,接收一个`std::string`类型的输入参数,代表需要分析的文本。
```cpp
#include <string>
#include <cctype>
void countCharacters(const std::string &text) {
int digitCount = 0;
int letterCount = 0;
int otherCount = 0; // 包括空格、标点等非字母数字字符
for (char c : text) {
if (isdigit(c)) { // 数字字符
digitCount++;
} else if (isalpha(c)) { // 字母字符
letterCount++;
} else { // 其他字符
otherCount++;
}
}
std::cout << "Number of digits: " << digitCount << '\n';
std::cout << "Number of letters: " << letterCount << '\n';
std::cout << "Number of other characters: " << otherCount << '\n';
}
```
2. 调用这个函数并传入你要分析的文本:
```cpp
int main() {
const std::string sampleText = "This is a sample text with 123 numbers and some special characters!@#";
countCharacters(sampleText);
return 0;
}
```
注意:这个代码会将所有非字母数字字符都算作“其他”类别。如果你想单独处理特定类型的特殊字符,你需要进一步修改代码以添加额外的条件判断。
阅读全文
相关推荐



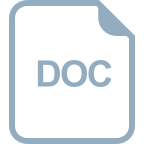



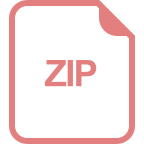








